Building C++ projects
The plugins described in this chapter are not compatible with the configuration cache. |
Gradle uses a convention-over-configuration approach to building native projects. If you are coming from another native build system, these concepts may be unfamiliar at first, but they serve a purpose to simplify build script authoring.
We will look at C++ projects in detail in this chapter, but most of the topics will apply to other supported native languages as well. If you don’t have much experience with building native projects with Gradle, take a look at the C++ tutorials for step-by-step instructions on how to build various types of basic C++ projects as well as some common use cases.
The C++ plugins covered in this chapter were introduced in 2018 and we recommend users to use those plugins over the older Native plugins that you may find references to.
Introduction
The simplest build script for a C++ project applies the C++ application plugin or the C++ library plugin and optionally sets the project version:
plugins {
`cpp-application` // or `cpp-library`
}
version = "1.2.1"
plugins {
id 'cpp-application' // or 'cpp-library'
}
version = '1.2.1'
By applying either of the C++ plugins, you get a whole host of features:
-
compileDebugCpp
andcompileReleaseCpp
tasks that compiles the C++ source files under src/main/cpp for the well-known debug and release build types, respectively. -
linkDebug
andlinkRelease
tasks that link the compiled C++ object files into an executable for applications or shared library for libraries with shared linkage for the debug and release build types. -
createDebug
andcreateRelease
tasks that assemble the compiled C++ object files into a static library for libraries with static linkage for the debug and release build types.
For any non-trivial C++ project, you’ll probably have some file dependencies and additional configuration specific to your project.
The C++ plugins also integrates the above tasks into the standard lifecycle tasks. The task that produces the development binary is attached to assemble
. By default, the development binary is the debug variant.
The rest of the chapter explains the different ways to customize the build to your requirements when building libraries and applications.
Introducing build variants
Native projects can typically produce several different binaries, such as debug or release ones, or ones that target particular platforms and processor architectures. Gradle manages this through the concepts of dimensions and variants.
A dimension is simply a category, where each category is orthogonal to the rest. For example, the "build type" dimension is a category that includes debug and release. The "architecture" dimension covers processor architectures like x86-64 and PowerPC.
A variant is a combination of values for these dimensions, consisting of exactly one value for each dimension. You might have a "debug x86-64" or a "release PowerPC" variant.
Gradle has built-in support for several dimensions and several values within each dimension. You can find a list of them in the native plugin reference chapter.
Declaring your source files
Gradle’s C++ support uses a ConfigurableFileCollection
directly from the application or library script block to configure the set of sources to compile.
Libraries make a distinction between private (implementation details) and public (exported to consumer) headers.
You can also configure sources for each binary build for those cases where sources are compiled only on certain target machines.
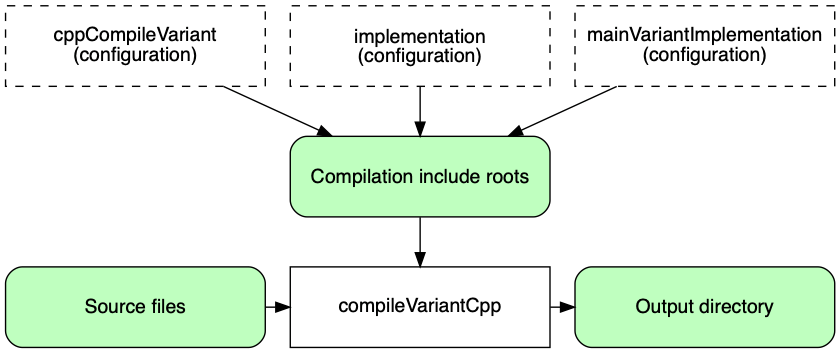
Test sources are configured on each test suite script block. See Testing C++ projects chapter.
Managing your dependencies
The vast majority of projects rely on other projects, so managing your project’s dependencies is an important part of building any project. Dependency management is a big topic, so we will only focus on the basics for C++ projects here. If you’d like to dive into the details, check out the introduction to dependency management.
Gradle provides support for consuming pre-built binaries from Maven repositories published by Gradle [1].
We will cover how to add dependencies between projects within a multi-build project.
Specifying dependencies for your C++ project requires two pieces of information:
-
Identifying information for the dependency (project path, Maven GAV)
-
What it’s needed for, e.g. compilation, linking, runtime or all of the above.
This information is specified in a dependencies {}
block of the C++ application
or library
script block.
For example, to tell Gradle that your project requires library common
to compile and link your production code, you can use the following fragment:
application {
dependencies {
implementation(project(":common"))
}
}
application {
dependencies {
implementation project(':common')
}
}
The Gradle terminology for the three elements is as follows:
-
Configuration (ex:
implementation
) - a named collection of dependencies, grouped together for a specific goal such as compiling or linking a module -
Project reference (ex:
project(':common')
) - the project referenced by the specified path
You can find a more comprehensive glossary of dependency management terms here.
As far as configurations go, the main ones of interest are:
-
implementation
- used for compilation, linking and runtime -
cppCompileVariant
- for dependencies that are necessary to compile your production code but shouldn’t be part of the linking or runtime process -
nativeLinkVariant
- for dependencies that are necessary to link your code but shouldn’t be part of the compilation or runtime process -
nativeRuntimeVariant
- for dependencies that are necessary to run your component but shouldn’t be part of the compilation or linking process
You can learn more about these and how they relate to one another in the native plugin reference chapter.
Be aware that the C++ Library Plugin creates an additional configuration — api
— for dependencies that are required for compiling and linking both the module and any modules that depend on it.
We have only scratched the surface here, so we recommend that you read the dedicated dependency management chapters once you’re comfortable with the basics of building C++ projects with Gradle.
Some common scenarios that require further reading include:
-
Defining a custom Maven-compatible repository
-
Declaring dependencies with changing (e.g. SNAPSHOT) and dynamic (range) versions
-
Declaring a sibling project as a dependency
-
Testing your fixes to 3rd-party dependency via composite builds (a better alternative to publishing to and consuming from Maven Local)
You’ll discover that Gradle has a rich API for working with dependencies — one that takes time to master, but is straightforward to use for common scenarios.
Compiling and linking your code
Compiling both your code can be trivially easy if you follow the conventions:
-
Put your source code under the src/main/cpp directory
-
Declare your compile dependencies in the
implementation
configurations (see the previous section) -
Run the
assemble
task
We recommend that you follow these conventions wherever possible, but you don’t have to.
There are several options for customization, as you’ll see next.
All CppCompile tasks are incremental and cacheable. |
Supported tool chain
Gradle offers the ability to execute the same build using different tool chains. When you build a native binary, Gradle will attempt to locate a tool chain installed on your machine that can build the binary. Gradle select the first tool chain that can build for the target operating system and architecture. In the future, Gradle will consider source and ABI compatibility when selecting a tool chain.
Gradle has general support for the three major tool chains on major operating system: Clang [2], GCC [3] and Visual C++ [4] (Windows-only). GCC and Clang installed using Macports and Homebrew have been reported to work fine, but this isn’t tested continuously.
Windows
To build on Windows, install a compatible version of Visual Studio. The C++ plugins will discover the Visual Studio installations and select the latest version. There is no need to mess around with environment variables or batch scripts. This works fine from a Cygwin shell or the Windows command-line.
Alternatively, you can install Cygwin or MinGW with GCC. Clang is currently not supported.
macOS
To build on macOS, you should install Xcode. The C++ plugins will discover the Xcode installation using the system PATH.
The C++ plugins also work with GCC and Clang installed with Macports or Homebrew [5]. To use one of the Macports or Homebrew, you will need to add Macports/Homebrew to the system PATH.
Linux
To build on Linux, install a compatible version of GCC or Clang. The C++ plugins will discover GCC or Clang using the system PATH.
Customizing file and directory locations
Imagine you have a legacy library project that uses an src directory for the production code and private headers and include directory for exported headers.
The conventional directory structure won’t work, so you need to tell Gradle where to find the source and header files.
You do that via the application
or library
script block.
Each component script block, as well as each binary, defines where it’s source code resides. You can override the convention values by using the following syntax:
library {
source.from(file("src"))
privateHeaders.from(file("src"))
publicHeaders.from(file("include"))
}
library {
source.from file('src')
privateHeaders.from file('src')
publicHeaders.from file('include')
}
Now Gradle will only search directly in src for the source and private headers and in include for public headers.
Changing compiler and linker options
Most of the compiler and linker options are accessible through the corresponding task, such as compileVariantCpp
, linkVariant
and createVariant
.
These tasks are of type CppCompile, LinkSharedLibrary and CreateStaticLibrary respectively.
Read the task reference for an up-to-date and comprehensive list of the options.
For example, if you want to change the warning level generated by the compiler for all variants, you can use this configuration:
tasks.withType(CppCompile::class.java).configureEach {
// Define a preprocessor macro for every binary
macros.put("NDEBUG", null)
// Define a compiler options
compilerArgs.add("-W3")
// Define toolchain-specific compiler options
compilerArgs.addAll(toolChain.map { toolChain ->
when (toolChain) {
is Gcc, is Clang -> listOf("-O2", "-fno-access-control")
is VisualCpp -> listOf("/Zi")
else -> listOf()
}
})
}
tasks.withType(CppCompile).configureEach {
// Define a preprocessor macro for every binary
macros.put("NDEBUG", null)
// Define a compiler options
compilerArgs.add '-W3'
// Define toolchain-specific compiler options
compilerArgs.addAll toolChain.map { toolChain ->
if (toolChain in [ Gcc, Clang ]) {
return ['-O2', '-fno-access-control']
} else if (toolChain in VisualCpp) {
return ['/Zi']
}
return []
}
}
It’s also possible to find the instance for a specific variant through the BinaryCollection
on the application
or library
script block:
application {
binaries.configureEach(CppStaticLibrary::class.java) {
// Define a preprocessor macro for every binary
compileTask.get().macros.put("NDEBUG", null)
// Define a compiler options
compileTask.get().compilerArgs.add("-W3")
// Define toolchain-specific compiler options
when (toolChain) {
is Gcc, is Clang -> compileTask.get().compilerArgs.addAll(listOf("-O2", "-fno-access-control"))
is VisualCpp -> compileTask.get().compilerArgs.add("/Zi")
}
}
}
application {
binaries.configureEach(CppStaticLibrary) {
// Define a preprocessor macro for every binary
compileTask.get().macros.put("NDEBUG", null)
// Define a compiler options
compileTask.get().compilerArgs.add '-W3'
// Define toolchain-specific compiler options
if (toolChain in [ Gcc, Clang ]) {
compileTask.get().compilerArgs.addAll(['-O2', '-fno-access-control'])
} else if (toolChain in VisualCpp) {
compileTask.get().compilerArgs.add('/Zi')
}
}
}
Selecting target machines
By default, Gradle will attempt to create a C++ binary variant for the host operating system and architecture.
It is possible to override this by specifying the set of TargetMachine
on the application
or library
script block:
application {
targetMachines = listOf(machines.windows.x86, machines.windows.x86_64, machines.macOS.x86_64, machines.linux.x86_64)
}
application {
targetMachines = [
machines.linux.x86_64,
machines.windows.x86, machines.windows.x86_64,
machines.macOS.x86_64
]
}
Packaging and publishing
How you package and potentially publish your C++ project varies greatly in the native world. Gradle comes with defaults, but custom packaging can be implemented without any issues.
-
Executable files are published directly to Maven repositories.
-
Shared and static library files are published directly to Maven repositories along with a zip of the public headers.
-
For applications, Gradle also supports installing and running the executable with all of its shared library dependencies in a known location.
Cleaning the build
The C++ Application and Library Plugins add a clean
task to you project by using the base plugin.
This task simply deletes everything in the layout.buildDirectory
directory, hence why you should always put files generated by the build in there.
The task is an instance of Delete and you can change what directory it deletes by setting its dir
property.
Building C++ libraries
The unique aspect of library projects is that they are used (or "consumed") by other C++ projects. That means the dependency metadata published with the binaries and headers — in the form of Gradle Module Metadata — is crucial. In particular, consumers of your library should be able to distinguish between two different types of dependencies: those that are only required to compile your library and those that are also required to compile the consumer.
Gradle manages this distinction via the C++ Library Plugin, which introduces an api configuration in addition to the implementation once covered in this chapter. If the types from a dependency appear as unresolved symbols of the static library or within the public headers then that dependency is exposed via your library’s public API and should, therefore, be added to the api configuration. Otherwise, the dependency is an internal implementation detail and should be added to implementation.
If you’re unsure of the difference between an API and implementation dependency, the C++ Library Plugin chapter has a detailed explanation. In addition, you can see a basic, practical example of building a C++ library in the corresponding sample.
Building C++ applications
See the C++ Application Plugin chapter for more details, but here’s a quick summary of what you get:
-
install
create a directory containing everything needed to run it -
Shell and Windows Batch scripts to start the application
You can see a basic example of building a C++ application in the corresponding sample.