Publishing Plugins to the Gradle Plugin Portal
Publishing a plugin is the primary way to make it available for others to use. While you can publish to a private repository to restrict access, publishing to the Gradle Plugin Portal makes your plugin available to anyone in the world.
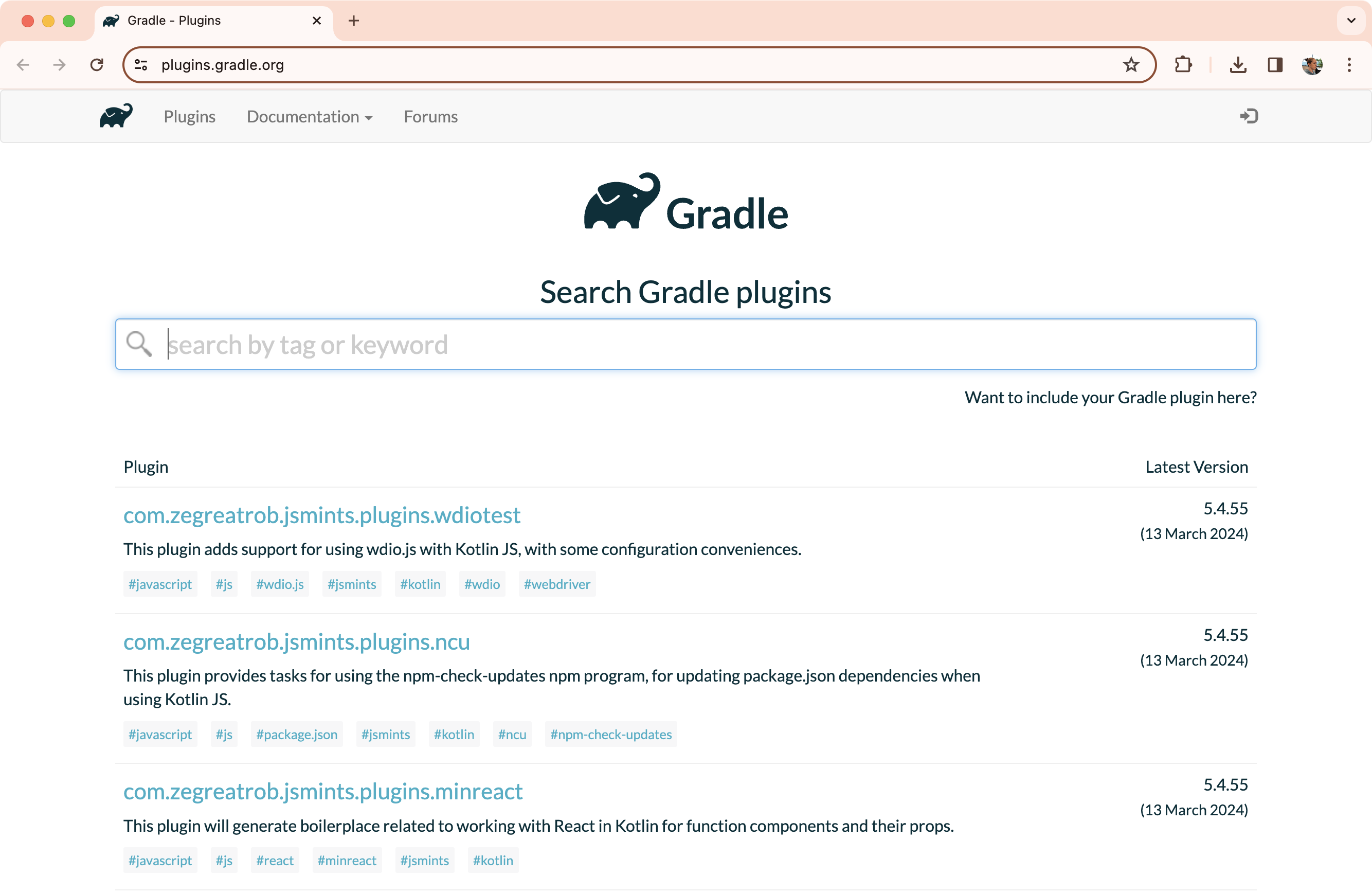
This guide shows you how to use the com.gradle.plugin-publish
plugin to publish plugins to the Gradle Plugin Portal using a convenient DSL.
This approach streamlines configuration steps and provides validation checks to ensure your plugin meets the Gradle Plugin Portal’s criteria.
Prerequisites
You’ll need an existing Gradle plugin project for this tutorial. If you don’t have one, use the Greeting plugin sample.
Attempting to publish this plugin will safely fail with a permission error, so don’t worry about cluttering up the Gradle Plugin Portal with a trivial example plugin.
Account setup
Before publishing your plugin, you must create an account on the Gradle Plugin Portal. Follow the instructions on the registration page to create an account and obtain an API key from your profile page’s "API Keys" tab.
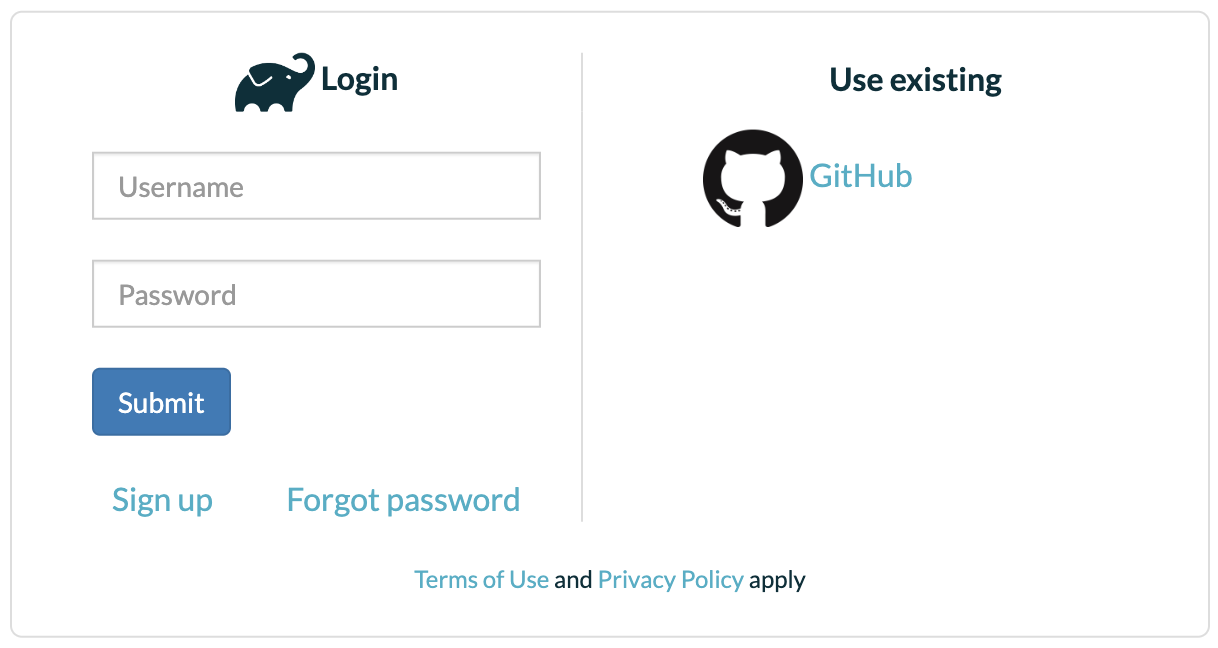
Store your API key in your Gradle configuration (gradle.publish.key and gradle.publish.secret) or use a plugin like Seauc Credentials plugin or Gradle Credentials plugin for secure management.
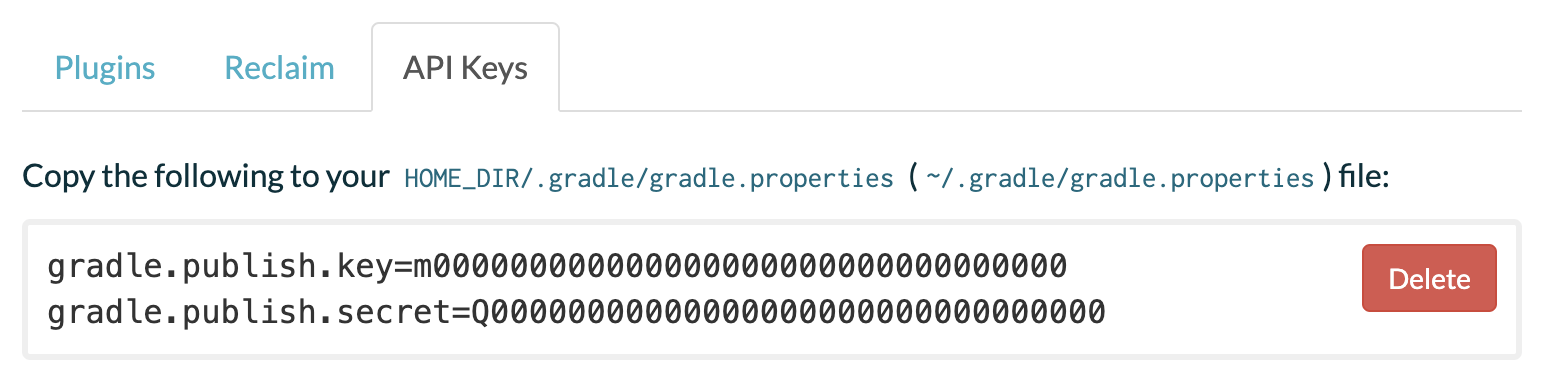
It is common practice to copy and paste the text into your $HOME/.gradle/gradle.properties file, but you can also place it in any other valid location.
All the plugin requires is that the gradle.publish.key
and gradle.publish.secret
are available as project properties when the appropriate Plugin Portal tasks are executed.
If you are concerned about placing your credentials in gradle.properties
, check out the Seauc Credentials plugin or the Gradle Credentials plugin.
Alternatively, you can provide the API key via GRADLE_PUBLISH_KEY
and GRADLE_PUBLISH_SECRET
environment variables.
This approach might be useful for CI/CD pipelines.
Adding the Plugin Publishing Plugin
To publish your plugin, add the com.gradle.plugin-publish
plugin to your project’s build.gradle
or build.gradle.kts
file:
plugins {
id("com.gradle.plugin-publish") version "1.2.1"
}
plugins {
id 'com.gradle.plugin-publish' version '1.2.1'
}
The latest version of the Plugin Publishing Plugin can be found on the Gradle Plugin Portal.
Since version 1.0.0 the Plugin Publish Plugin automatically applies the Java Gradle Plugin Development Plugin (assists with developing Gradle plugins) and the Maven Publish Plugin (generates plugin publication metadata). If using older versions of the Plugin Publish Plugin, these helper plugins must be applied explicitly. |
Configuring the Plugin Publishing Plugin
Configure the com.gradle.plugin-publish
plugin in your build.gradle
or build.gradle.kts
file.
group = "io.github.johndoe" (1)
version = "1.0" (2)
gradlePlugin { (3)
website = "<substitute your project website>" (4)
vcsUrl = "<uri to project source repository>" (5)
// ... (6)
}
group = 'io.github.johndoe' (1)
version = '1.0' (2)
gradlePlugin { (3)
website = '<substitute your project website>' (4)
vcsUrl = '<uri to project source repository>' (5)
// ... (6)
}
1 | Make sure your project has a group set which is used to identify the artifacts (jar and metadata) you publish for your plugins
in the repository of the Gradle Plugin Portal and which is descriptive of the plugin author or the organization the plugins belong too. |
2 | Set the version of your project, which will also be used as the version of your plugins. |
3 | Use the gradlePlugin block provided by the Java Gradle Plugin Development Plugin
to configure further options for your plugin publication. |
4 | Set the website for your plugin’s project. |
5 | Provide the source repository URI so that others can find it, if they want to contribute. |
6 | Set specific properties for each plugin you want to publish; see next section. |
Define common properties for all plugins, such as group, version, website, and source repository, using the gradlePlugin{}
block:
gradlePlugin { (1)
// ... (2)
plugins { (3)
create("greetingsPlugin") { (4)
id = "<your plugin identifier>" (5)
displayName = "<short displayable name for plugin>" (6)
description = "<human-readable description of what your plugin is about>" (7)
tags = listOf("tags", "for", "your", "plugins") (8)
implementationClass = "<your plugin class>"
}
}
}
gradlePlugin { (1)
// ... (2)
plugins { (3)
greetingsPlugin { (4)
id = '<your plugin identifier>' (5)
displayName = '<short displayable name for plugin>' (6)
description = '<human-readable description of what your plugin is about>' (7)
tags.set(['tags', 'for', 'your', 'plugins']) (8)
implementationClass = '<your plugin class>'
}
}
}
1 | Plugin specific configuration also goes into the gradlePlugin block. |
2 | This is where we previously added global properties. |
3 | Each plugin you publish will have its own block inside plugins . |
4 | The name of a plugin block must be unique for each plugin you publish; this is a property used only locally by your build and will not be part of the publication. |
5 | Set the unique id of the plugin, as it will be identified in the publication. |
6 | Set the plugin name in human-readable form. |
7 | Set a description to be displayed on the portal. It provides useful information to people who want to use your plugin. |
8 | Specifies the categories your plugin covers. It makes the plugin more likely to be discovered by people needing its functionality. |
For example, consider the configuration for the GradleTest plugin, already published to the Gradle Plugin Portal.
gradlePlugin {
website = "https://github.com/ysb33r/gradleTest"
vcsUrl = "https://github.com/ysb33r/gradleTest.git"
plugins {
create("gradletestPlugin") {
id = "org.ysb33r.gradletest"
displayName = "Plugin for compatibility testing of Gradle plugins"
description = "A plugin that helps you test your plugin against a variety of Gradle versions"
tags = listOf("testing", "integrationTesting", "compatibility")
implementationClass = "org.ysb33r.gradle.gradletest.GradleTestPlugin"
}
}
}
gradlePlugin {
website = 'https://github.com/ysb33r/gradleTest'
vcsUrl = 'https://github.com/ysb33r/gradleTest.git'
plugins {
gradletestPlugin {
id = 'org.ysb33r.gradletest'
displayName = 'Plugin for compatibility testing of Gradle plugins'
description = 'A plugin that helps you test your plugin against a variety of Gradle versions'
tags.addAll('testing', 'integrationTesting', 'compatibility')
implementationClass = 'org.ysb33r.gradle.gradletest.GradleTestPlugin'
}
}
}
If you browse the associated page on the Gradle Plugin Portal for the GradleTest plugin, you will see how the specified metadata is displayed.
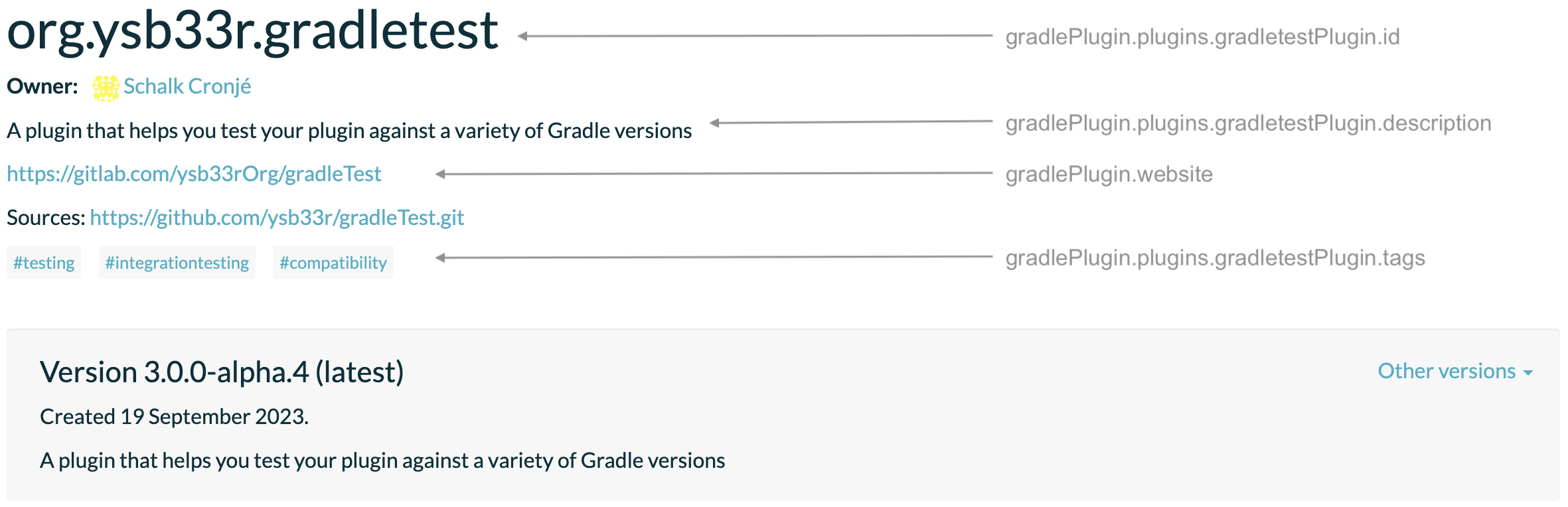
Sources & Javadoc
The Plugin Publish Plugin automatically generates and publishes the Javadoc, and sources JARs for your plugin publication.
Sign artifacts
Starting from version 1.0.0 of Plugin Publish Plugin, the signing of published plugin artifacts has been made automatic.
To enable it, all that’s needed is to apply the signing
plugin in your build.
Shadow dependencies
Starting from version 1.0.0 of Plugin Publish Plugin, shadowing your plugin’s dependencies (ie, publishing it as a fat jar) has been made automatic.
To enable it, all that’s needed is to apply the com.gradleup.shadow
plugin in your build.
Publishing the plugin
If you publish your plugin internally for use within your organization, you can publish it like any other code artifact. See the Ivy and Maven chapters on publishing artifacts.
If you are interested in publishing your plugin to be used by the wider Gradle community, you can publish it to Gradle Plugin Portal. This site provides the ability to search for and gather information about plugins contributed by the Gradle community. Please refer to the corresponding section on making your plugin available on this site.
Publish locally
To check how the artifacts of your published plugin look or to use it only locally or internally in your company, you can publish it to any Maven repository, including a local folder.
You only need to configure repositories for publishing.
Then, you can run the publish
task to publish your plugin to all repositories you have defined (but not the Gradle Plugin Portal).
publishing {
repositories {
maven {
name = "localPluginRepository"
url = uri("../local-plugin-repository")
}
}
}
publishing {
repositories {
maven {
name = 'localPluginRepository'
url = file('../local-plugin-repository')
}
}
}
To use the repository in another build, add it to the repositories of the pluginManagement {}
block in your settings.gradle(.kts)
file.
Publish to the Plugin Portal
Publish the plugin by using the publishPlugin
task:
$ ./gradlew publishPlugins
You can validate your plugins before publishing using the --validate-only
flag:
$ ./gradlew publishPlugins --validate-only
If you have not configured your gradle.properties
for the Gradle Plugin Portal, you can specify them on the command-line:
$ ./gradlew publishPlugins -Pgradle.publish.key=<key> -Pgradle.publish.secret=<secret>
You will encounter a permission failure if you attempt to publish the example Greeting Plugin with the ID used in this section. That’s expected and ensures the portal won’t be overrun with multiple experimental and duplicate greeting-type plugins. |
After approval, your plugin will be available on the Gradle Plugin Portal for others to discover and use.
Consume the published plugin
Once you successfully publish a plugin, it won’t immediately appear on the Portal. It also needs to pass an approval process, which is manual and relatively slow for the initial version of your plugin, but is fully automatic for subsequent versions. For further details, see here.
Once your plugin is approved, you can find instructions for its use at a URL of the form https://plugins.gradle.org/plugin/<your-plugin-id>. For example, the Greeting Plugin example is already on the portal at https://plugins.gradle.org/plugin/org.example.greeting.
Plugins published without Gradle Plugin Portal
If your plugin was published without using the Java Gradle Plugin Development Plugin, the publication will be lacking Plugin Marker Artifact, which is needed for plugins DSL to locate the plugin.
In this case, the recommended way to resolve the plugin in another project is to add a resolutionStrategy
section to the pluginManagement {}
block of the project’s settings file, as shown below.
resolutionStrategy {
eachPlugin {
if (requested.id.namespace == "org.example") {
useModule("org.example:custom-plugin:${requested.version}")
}
}
}
resolutionStrategy {
eachPlugin {
if (requested.id.namespace == 'org.example') {
useModule("org.example:custom-plugin:${requested.version}")
}
}
}