The Groovy Plugin
The Groovy plugin extends the Java plugin to add support for Groovy projects. It can deal with Groovy code, mixed Groovy and Java code, and even pure Java code (although we don’t necessarily recommend to use it for the latter). The plugin supports joint compilation, which allows you to freely mix and match Groovy and Java code, with dependencies in both directions. For example, a Groovy class can extend a Java class that in turn extends a Groovy class. This makes it possible to use the best language for the job, and to rewrite any class in the other language if needed.
Note that if you want to benefit from the API / implementation separation, you can also apply the java-library
plugin to your Groovy project.
Usage
To use the Groovy plugin, include the following in your build script:
plugins {
groovy
}
plugins {
id 'groovy'
}
Tasks
The Groovy plugin adds the following tasks to the project. Information about altering the dependencies to Java compile tasks are found here.
compileGroovy
— GroovyCompile-
Depends on:
compileJava
Compiles production Groovy source files.
compileTestGroovy
— GroovyCompile-
Depends on:
compileTestJava
Compiles test Groovy source files.
compileSourceSetGroovy
— GroovyCompile-
Depends on:
compileSourceSetJava
Compiles the given source set’s Groovy source files.
groovydoc
— Groovydoc-
Generates API documentation for the production Groovy source files.
The Groovy plugin adds the following dependencies to tasks added by the Java plugin.
Task name | Depends on |
---|---|
|
|
|
|
|
|
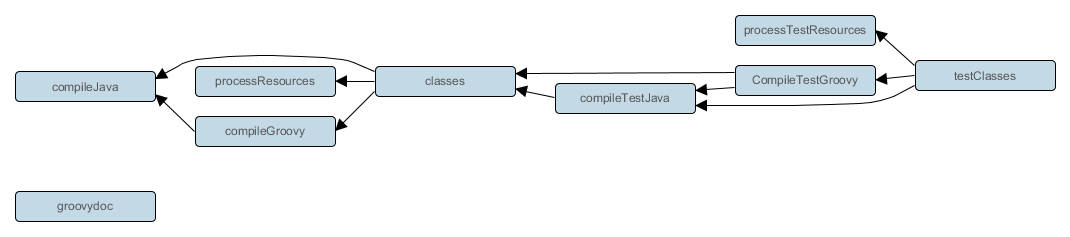
Project layout
The Groovy plugin assumes the project layout shown in Groovy Layout. All the Groovy source directories can contain Groovy and Java code. The Java source directories may only contain Java source code.[1] None of these directories need to exist or have anything in them; the Groovy plugin will simply compile whatever it finds.
src/main/java
-
Production Java source.
src/main/resources
-
Production resources, such as XML and properties files.
src/main/groovy
-
Production Groovy source. May also contain Java source files for joint compilation.
src/test/java
-
Test Java source.
src/test/resources
-
Test resources.
src/test/groovy
-
Test Groovy source. May also contain Java source files for joint compilation.
src/sourceSet/java
-
Java source for the source set named sourceSet.
src/sourceSet/resources
-
Resources for the source set named sourceSet.
src/sourceSet/groovy
-
Groovy source files for the given source set. May also contain Java source files for joint compilation.
Changing the project layout
Just like the Java plugin, the Groovy plugin allows you to configure custom locations for Groovy production and test source files.
sourceSets {
main {
groovy {
setSrcDirs(listOf("src/groovy"))
}
}
test {
groovy {
setSrcDirs(listOf("test/groovy"))
}
}
}
sourceSets {
main {
groovy {
srcDirs = ['src/groovy']
}
}
test {
groovy {
srcDirs = ['test/groovy']
}
}
}
Dependency management
Because Gradle’s build language is based on Groovy, and parts of Gradle are implemented in Groovy, Gradle already ships with a Groovy library. Nevertheless, Groovy projects need to explicitly declare a Groovy dependency. This dependency will then be used on compile and runtime class paths. It will also be used to get hold of the Groovy compiler and Groovydoc tool, respectively.
If Groovy is used for production code, the Groovy dependency should be added to the implementation
configuration:
repositories {
mavenCentral()
}
dependencies {
implementation("org.codehaus.groovy:groovy-all:2.4.15")
}
repositories {
mavenCentral()
}
dependencies {
implementation 'org.codehaus.groovy:groovy-all:2.4.15'
}
If Groovy is only used for test code, the Groovy dependency should be added to the testImplementation
configuration:
dependencies {
testImplementation("org.codehaus.groovy:groovy-all:2.4.15")
}
dependencies {
testImplementation 'org.codehaus.groovy:groovy-all:2.4.15'
}
To use the Groovy library that ships with Gradle, declare a localGroovy()
dependency. Note that different Gradle versions ship with different Groovy versions; as such, using localGroovy()
is less safe then declaring a regular Groovy dependency.
dependencies {
implementation(localGroovy())
}
dependencies {
implementation localGroovy()
}
Automatic configuration of groovyClasspath
The GroovyCompile
and Groovydoc
tasks consume Groovy code in two ways: on their classpath
, and on their groovyClasspath
. The former is used to locate classes referenced by the source code, and will typically contain the Groovy library along with other libraries. The latter is used to load and execute the Groovy compiler and Groovydoc tool, respectively, and should only contain the Groovy library and its dependencies.
Unless a task’s groovyClasspath
is configured explicitly, the Groovy (base) plugin will try to infer it from the task’s classpath
. This is done as follows:
-
If a
groovy-all(-indy)
Jar is found onclasspath
, that jar will be added togroovyClasspath
. -
If a
groovy(-indy)
jar is found onclasspath
, and the project has at least one repository declared, a correspondinggroovy(-indy)
repository dependency will be added togroovyClasspath
. -
Otherwise, execution of the task will fail with a message saying that
groovyClasspath
could not be inferred.
Note that the “-indy
” variation of each jar refers to the version with invokedynamic
support.
Convention properties
The Groovy plugin does not add any convention properties to the project.
Source set properties
The Groovy plugin adds the following extensions to each source set in the project. You can use these properties in your build script as though they were properties of the source set object.
Groovy Plugin — source set properties
groovy
— GroovySourceDirectorySet (read-only)-
Default value: Not null
The Groovy source files of this source set. Contains all
.groovy
and.java
files found in the Groovy source directories, and excludes all other types of files. groovy.srcDirs
—Set<File>
-
Default value:
[projectDir/src/name/groovy]
The source directories containing the Groovy source files of this source set. May also contain Java source files for joint compilation. Can set using anything described in Specifying Multiple Files.
allGroovy
— FileTree (read-only)-
Default value: Not null
All Groovy source files of this source set. Contains only the
.groovy
files found in the Groovy source directories.
These properties are provided by a convention object of type GroovySourceSet.
The Groovy plugin also modifies some source set properties:
Groovy Plugin - modified source set properties
Property name | Change |
---|---|
|
Adds all |
|
Adds all source files found in the Groovy source directories. |
GroovyCompile
The Groovy plugin adds a GroovyCompile task for each source set in the project.
The task type shares much with the JavaCompile
task by extending AbstractCompile
(see the relevant Java Plugin section).
The GroovyCompile
task supports most configuration options of the official Groovy compiler.
The task can also leverage the Java toolchain support.
Task Property | Type | Default Value |
---|---|---|
|
|
|
|
FileTree. Can set using anything described in Specifying Multiple Files. |
|
|
|
|
|
|
|
|
|
None but will be configured if a toolchain is defined on the |
Compilation avoidance
Caveat: Groovy compilation avoidance is an incubating feature since Gradle 5.6. There are known inaccuracies so please enable it at your own risk.
To enable the incubating support for Groovy compilation avoidance, add a enableFeaturePreview
to your settings file:
enableFeaturePreview('GROOVY_COMPILATION_AVOIDANCE')
enableFeaturePreview("GROOVY_COMPILATION_AVOIDANCE")
If a dependent project has changed in an ABI-compatible way (only its private API has changed), then Groovy compilation tasks will be up-to-date.
This means that if project A
depends on project B
and a class in B
is changed in an ABI-compatible way (typically, changing only the body of a method), then Gradle won’t recompile A
.
See Java compile avoidance for a detailed list of the types of changes that do not affect the ABI and are ignored.
However, similar to Java’s annotation processing, there are various ways to customize the Groovy compilation process, for which implementation details matter.
Some well-known examples are Groovy AST transformations.
In these cases, these dependencies must be declared separately in a classpath called astTransformationClasspath
:
val astTransformation by configurations.creating
dependencies {
astTransformation(project(":ast-transformation"))
}
tasks.withType<GroovyCompile>().configureEach {
astTransformationClasspath.from(astTransformation)
}
configurations { astTransformation }
dependencies {
astTransformation(project(":ast-transformation"))
}
tasks.withType(GroovyCompile).configureEach {
astTransformationClasspath.from(configurations.astTransformation)
}
Incremental Groovy compilation
Since 5.6, Gradle introduces an experimental incremental Groovy compiler. To enable incremental compilation for Groovy, you need:
-
Enable Groovy compilation avoidance.
-
Explicitly enable incremental Groovy compilation in the build script:
tasks.withType<GroovyCompile>().configureEach {
options.isIncremental = true
options.incrementalAfterFailure = true
}
tasks.withType(GroovyCompile).configureEach {
options.incremental = true
options.incrementalAfterFailure = true
}
This gives you the following benefits:
-
Incremental builds are much faster.
-
If only a small set of Groovy source files are changed, only the affected source files will be recompiled. Classes that don’t need to be recompiled remain unchanged in the output directory. For example, if you only change a few Groovy test classes, you don’t need to recompile all Groovy test source files — only the changed ones need to be recompiled.
To understand how incremental compilation works, see Incremental Java compilation for a detailed overview. Note that there’re several differences from Java incremental compilation:
The Groovy compiler doesn’t keep @Retention
in generated annotation class bytecode (GROOVY-9185), thus all annotations are RUNTIME
.
This means that changes to source-retention annotations won’t trigger a full recompilation.
Known issues
-
Changes to resources won’t trigger a recompilation, this might result in some incorrectness — for example Extension Modules.
Compiling and testing for Java 6 or Java 7
With toolchain support added to GroovyCompile
, it is possible to compile Groovy code using a different Java version than the one running Gradle.
If you also have Java source files, this will also configure JavaCompile
to use the right Java compiler is used, as can be seen in the Java plugin documentation.
Example: Configure Java 7 build for Groovy
java {
toolchain {
languageVersion = JavaLanguageVersion.of(7)
}
}
java {
toolchain {
languageVersion = JavaLanguageVersion.of(7)
}
}