Declaring repositories
- Declaring a publicly-available repository
- Declaring a custom repository by URL
- Declaring multiple repositories
- Supported repository types
- Maven repositories
- The case for mavenLocal()
- Ivy repositories
- Repository content filtering
- Supported metadata sources
- Plugin repositories vs. build repositories
- Centralizing repositories declaration
- Supported repository transport protocols
- HTTP(S) authentication schemes configuration
- AWS S3 repositories configuration
- Google Cloud Storage repositories configuration
- Handling credentials
Gradle can resolve dependencies from one or many repositories based on Maven, Ivy or flat directory formats. Check out the full reference on all types of repositories for more information.
Declaring a publicly-available repository
Organizations building software may want to leverage public binary repositories to download and consume open source dependencies. Popular public repositories include Maven Central and the Google Android repository. Gradle provides built-in shorthand notations for these widely-used repositories.
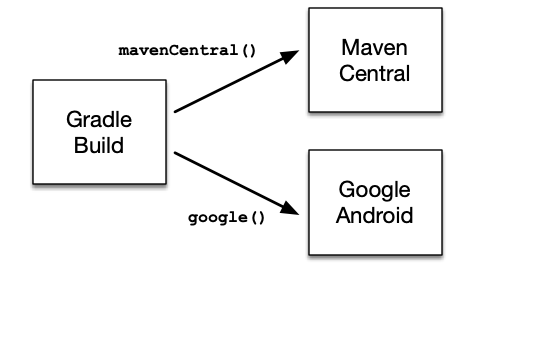
Under the covers Gradle resolves dependencies from the respective URL of the public repository defined by the shorthand notation. All shorthand notations are available via the RepositoryHandler API. Alternatively, you can spell out the URL of the repository for more fine-grained control.
Maven Central repository
Maven Central is a popular repository hosting open source libraries for consumption by Java projects.
To declare the Maven Central repository for your build add this to your script:
repositories {
mavenCentral()
}
repositories {
mavenCentral()
}
Google Maven repository
The Google repository hosts Android-specific artifacts including the Android SDK. For usage examples, see the relevant Android documentation.
To declare the Google Maven repository add this to your build script:
repositories {
google()
}
repositories {
google()
}
Declaring a custom repository by URL
Most enterprise projects set up a binary repository available only within an intranet. In-house repositories enable teams to publish internal binaries, setup user management and security measure and ensure uptime and availability. Specifying a custom URL is also helpful if you want to declare a less popular, but publicly-available repository.
Repositories with custom URLs can be specified as Maven or Ivy repositories by calling the corresponding methods available on the RepositoryHandler API.
Gradle supports other protocols than http
or https
as part of the custom URL e.g. file
, sftp
or s3
.
For a full coverage see the section on supported repository types.
You can also define your own repository layout by using ivy { }
repositories as they are very flexible in terms of how modules are organised in a repository.
Declaring multiple repositories
You can define more than one repository for resolving dependencies. Declaring multiple repositories is helpful if some dependencies are only available in one repository but not the other. You can mix any type of repository described in the reference section.
This example demonstrates how to declare various named and custom URL repositories for a project:
repositories {
mavenCentral()
maven {
url = uri("https://repo.spring.io/release")
}
maven {
url = uri("https://repository.jboss.org/maven2")
}
}
repositories {
mavenCentral()
maven {
url "https://repo.spring.io/release"
}
maven {
url "https://repository.jboss.org/maven2"
}
}
The order of declaration determines how Gradle will check for dependencies at runtime. If Gradle finds a module descriptor in a particular repository, it will attempt to download all of the artifacts for that module from the same repository. You can learn more about the inner workings of dependency downloads. |
Strict limitation to declared repositories
Maven POM metadata can reference additional repositories. These will be ignored by Gradle, which will only use the repositories declared in the build itself.
This is a reproducibility safe-guard but also a security protection. Without it, an updated version of a dependency could pull artifacts from anywhere into your build. |
Supported repository types
Gradle supports a wide range of sources for dependencies, both in terms of format and in terms of connectivity. You may resolve dependencies from:
-
Different formats
-
a Maven compatible artifact repository (e.g: Maven Central)
-
an Ivy compatible artifact repository (including custom layouts)
-
-
with different connectivity
-
a wide variety of remote protocols such as HTTPS, SFTP, AWS S3 and Google Cloud Storage
Flat directory repository
Some projects might prefer to store dependencies on a shared drive or as part of the project source code instead of a binary repository product. If you want to use a (flat) filesystem directory as a repository, simply type:
repositories {
flatDir {
dirs("lib")
}
flatDir {
dirs("lib1", "lib2")
}
}
repositories {
flatDir {
dirs 'lib'
}
flatDir {
dirs 'lib1', 'lib2'
}
}
This adds repositories which look into one or more directories for finding dependencies.
This type of repository does not support any meta-data formats like Ivy XML or Maven POM files. Instead, Gradle will dynamically generate a module descriptor (without any dependency information) based on the presence of artifacts.
As Gradle prefers to use modules whose descriptor has been created from real meta-data rather than being generated, flat directory repositories cannot be used to override artifacts with real meta-data from other repositories declared in the build. For example, if Gradle finds only For the use case of overriding remote artifacts with local ones consider using an Ivy or Maven repository instead whose URL points to a local directory. |
If you only work with flat directory repositories you don’t need to set all attributes of a dependency.
Local repositories
The following sections describe repositories format, Maven or Ivy. These can be declared as local repositories, using a local filesystem path to access them.
The difference with the flat directory repository is that they do respect a format and contain metadata.
When such a repository is configured, Gradle totally bypasses its dependency cache for it as there can be no guarantee that content may not change between executions. Because of that limitation, they can have a performance impact.
They also make build reproducibility much harder to achieve and their use should be limited to tinkering or prototyping.
Maven repositories
Many organizations host dependencies in an in-house Maven repository only accessible within the company’s network. Gradle can declare Maven repositories by URL.
For adding a custom Maven repository you can do:
repositories {
maven {
url = uri("http://repo.mycompany.com/maven2")
}
}
repositories {
maven {
url "http://repo.mycompany.com/maven2"
}
}
Setting up composite Maven repositories
Sometimes a repository will have the POMs published to one location, and the JARs and other artifacts published at another location. To define such a repository, you can do:
repositories {
maven {
// Look for POMs and artifacts, such as JARs, here
url = uri("http://repo2.mycompany.com/maven2")
// Look for artifacts here if not found at the above location
artifactUrls("http://repo.mycompany.com/jars")
artifactUrls("http://repo.mycompany.com/jars2")
}
}
repositories {
maven {
// Look for POMs and artifacts, such as JARs, here
url "http://repo2.mycompany.com/maven2"
// Look for artifacts here if not found at the above location
artifactUrls "http://repo.mycompany.com/jars"
artifactUrls "http://repo.mycompany.com/jars2"
}
}
Gradle will look at the base url
location for the POM and the JAR.
If the JAR can’t be found there, the extra artifactUrls
are used to look for JARs.
Accessing authenticated Maven repositories
You can specify credentials for Maven repositories secured by different type of authentication.
See Supported repository transport protocols for authentication options.
Local Maven repository
Gradle can consume dependencies available in the local Maven repository. Declaring this repository is beneficial for teams that publish to the local Maven repository with one project and consume the artifacts by Gradle in another project.
Gradle stores resolved dependencies in its own cache. A build does not need to declare the local Maven repository even if you resolve dependencies from a Maven-based, remote repository. |
Before adding Maven local as a repository, you should make sure this is really required. |
To declare the local Maven cache as a repository add this to your build script:
repositories {
mavenLocal()
}
repositories {
mavenLocal()
}
Gradle uses the same logic as Maven to identify the location of your local Maven cache.
If a local repository location is defined in a settings.xml
, this location will be used.
The settings.xml
in <home directory of the current user>/.m2
takes precedence over the settings.xml
in M2_HOME/conf
.
If no settings.xml
is available, Gradle uses the default location <home directory of the current user>/.m2/repository
.
The case for mavenLocal()
As a general advice, you should avoid adding mavenLocal()
as a repository.
There are different issues with using mavenLocal()
that you should be aware of:
-
Maven uses it as a cache, not a repository, meaning it can contain partial modules.
-
For example, if Maven never downloaded the source or javadoc files for a given module, Gradle will not find them either since it searches for files in a single repository once a module has been found.
-
-
As a local repository, Gradle does not trust its content, because:
-
Origin of artifacts cannot be tracked, which is a correctness and security problem
-
Artifacts can be easily overwritten, which is a security, correctness and reproducibility problem
-
-
To mitigate the fact that metadata and/or artifacts can be changed, Gradle does not perform any caching for local repositories
-
As a consequence, your builds are slower
-
Given that order of repositories is important, adding
mavenLocal()
first means that all your builds are going to be slower
-
There are a few cases where you might have to use mavenLocal()
:
-
For interoperability with Maven
-
For example, project A is built with Maven, project B is built with Gradle, and you need to share the artifacts during development
-
It is always preferable to use an internal full featured repository instead
-
In case this is not possible, you should limit this to local builds only
-
-
For interoperability with Gradle itself
-
In a multi-repository world, you want to check that changes to project A work with project B
-
It is preferable to use composite builds for this use case
-
If for some reason neither composite builds nor full featured repository are possible, then
mavenLocal()
is a last resort option
-
After all these warnings, if you end up using mavenLocal()
, consider combining it with a repository filter.
This will make sure it only provides what is expected and nothing else.
Ivy repositories
Organizations might decide to host dependencies in an in-house Ivy repository. Gradle can declare Ivy repositories by URL.
Defining an Ivy repository with a standard layout
To declare an Ivy repository using the standard layout no additional customization is needed. You just declare the URL.
repositories {
ivy {
url = uri("http://repo.mycompany.com/repo")
}
}
repositories {
ivy {
url "http://repo.mycompany.com/repo"
}
}
Defining a named layout for an Ivy repository
You can specify that your repository conforms to the Ivy or Maven default layout by using a named layout.
repositories {
ivy {
url = uri("http://repo.mycompany.com/repo")
layout("maven")
}
}
repositories {
ivy {
url "http://repo.mycompany.com/repo"
layout "maven"
}
}
Valid named layout values are 'gradle'
(the default), 'maven'
and 'ivy'
. See IvyArtifactRepository.layout(java.lang.String) in the API documentation for details of these named layouts.
Defining custom pattern layout for an Ivy repository
To define an Ivy repository with a non-standard layout, you can define a pattern layout for the repository:
repositories {
ivy {
url = uri("http://repo.mycompany.com/repo")
patternLayout {
artifact("[module]/[revision]/[type]/[artifact].[ext]")
}
}
}
repositories {
ivy {
url "http://repo.mycompany.com/repo"
patternLayout {
artifact "[module]/[revision]/[type]/[artifact].[ext]"
}
}
}
To define an Ivy repository which fetches Ivy files and artifacts from different locations, you can define separate patterns to use to locate the Ivy files and artifacts:
Each artifact
or ivy
specified for a repository adds an additional pattern to use. The patterns are used in the order that they are defined.
repositories {
ivy {
url = uri("http://repo.mycompany.com/repo")
patternLayout {
artifact("3rd-party-artifacts/[organisation]/[module]/[revision]/[artifact]-[revision].[ext]")
artifact("company-artifacts/[organisation]/[module]/[revision]/[artifact]-[revision].[ext]")
ivy("ivy-files/[organisation]/[module]/[revision]/ivy.xml")
}
}
}
repositories {
ivy {
url "http://repo.mycompany.com/repo"
patternLayout {
artifact "3rd-party-artifacts/[organisation]/[module]/[revision]/[artifact]-[revision].[ext]"
artifact "company-artifacts/[organisation]/[module]/[revision]/[artifact]-[revision].[ext]"
ivy "ivy-files/[organisation]/[module]/[revision]/ivy.xml"
}
}
}
Optionally, a repository with pattern layout can have its 'organisation'
part laid out in Maven style, with forward slashes replacing dots as separators. For example, the organisation my.company
would then be represented as my/company
.
repositories {
ivy {
url = uri("http://repo.mycompany.com/repo")
patternLayout {
artifact("[organisation]/[module]/[revision]/[artifact]-[revision].[ext]")
setM2compatible(true)
}
}
}
repositories {
ivy {
url "http://repo.mycompany.com/repo"
patternLayout {
artifact "[organisation]/[module]/[revision]/[artifact]-[revision].[ext]"
m2compatible = true
}
}
}
Accessing authenticated Ivy repositories
You can specify credentials for Ivy repositories secured by basic authentication.
repositories {
ivy {
url = uri("http://repo.mycompany.com")
credentials {
username = "user"
password = "password"
}
}
}
repositories {
ivy {
url "http://repo.mycompany.com"
credentials {
username "user"
password "password"
}
}
}
See Supported repository transport protocols for authentication options.
Repository content filtering
Gradle exposes an API to declare what a repository may or may not contain. There are different use cases for it:
-
performance, when you know a dependency will never be found in a specific repository
-
security, by avoiding leaking what dependencies are used in a private project
-
reliability, when some repositories contain corrupted metadata or artifacts
It’s even more important when considering that the declared order of repositories matter.
Declaring a repository filter
repositories {
maven {
url = uri("https://repo.mycompany.com/maven2")
content {
// this repository *only* contains artifacts with group "my.company"
includeGroup("my.company")
}
}
mavenCentral {
content {
// this repository contains everything BUT artifacts with group starting with "my.company"
excludeGroupByRegex("my\\.company.*")
}
}
}
repositories {
maven {
url "https://repo.mycompany.com/maven2"
content {
// this repository *only* contains artifacts with group "my.company"
includeGroup "my.company"
}
}
mavenCentral {
content {
// this repository contains everything BUT artifacts with group starting with "my.company"
excludeGroupByRegex "my\\.company.*"
}
}
}
By default, repositories include everything and exclude nothing:
-
If you declare an include, then it excludes everything but what is included.
-
If you declare an exclude, then it includes everything but what is excluded.
-
If you declare both includes and excludes, then it includes only what is explicitly included and not excluded.
It is possible to filter either by explicit group, module or version, either strictly or using regular expressions. When using a strict version, it is possible to use a version range, using the format supported by Gradle. In addition, there are filtering options by resolution context: configuration name or even configuration attributes. See RepositoryContentDescriptor for details.
Declaring content exclusively found in one repository
Filters declared using the repository-level content filter are not exclusive. This means that declaring that a repository includes an artifact doesn’t mean that the other repositories can’t have it either: you must declare what every repository contains in extension.
Alternatively, Gradle provides an API which lets you declare that a repository exclusively includes an artifact. If you do so:
-
an artifact declared in a repository can’t be found in any other
-
exclusive repository content must be declared in extension (just like for repository-level content)
repositories {
// This repository will _not_ be searched for artifacts in my.company
// despite being declared first
mavenCentral()
exclusiveContent {
forRepository {
maven {
url = uri("https://repo.mycompany.com/maven2")
}
}
filter {
// this repository *only* contains artifacts with group "my.company"
includeGroup("my.company")
}
}
}
repositories {
// This repository will _not_ be searched for artifacts in my.company
// despite being declared first
mavenCentral()
exclusiveContent {
forRepository {
maven {
url "https://repo.mycompany.com/maven2"
}
}
filter {
// this repository *only* contains artifacts with group "my.company"
includeGroup "my.company"
}
}
}
It is possible to filter either by explicit group, module or version, either strictly or using regular expressions. See InclusiveRepositoryContentDescriptor for details.
If you leverage exclusive content filtering in the Your options are either to declare all repositories in settings or to use non-exclusive content filtering. |
Maven repository filtering
For Maven repositories, it’s often the case that a repository would either contain releases or snapshots. Gradle lets you declare what kind of artifacts are found in a repository using this DSL:
repositories {
maven {
url = uri("https://repo.mycompany.com/releases")
mavenContent {
releasesOnly()
}
}
maven {
url = uri("https://repo.mycompany.com/snapshots")
mavenContent {
snapshotsOnly()
}
}
}
repositories {
maven {
url "https://repo.mycompany.com/releases"
mavenContent {
releasesOnly()
}
}
maven {
url "https://repo.mycompany.com/snapshots"
mavenContent {
snapshotsOnly()
}
}
}
Supported metadata sources
When searching for a module in a repository, Gradle, by default, checks for supported metadata file formats in that repository.
In a Maven repository, Gradle looks for a .pom
file, in an ivy repository it looks for an ivy.xml
file and in a flat directory repository it looks directly for .jar
files as it does not expect any metadata.
Starting with 5.0, Gradle also looks for .module
(Gradle module metadata) files.
However, if you define a customized repository you might want to configure this behavior.
For example, you can define a Maven repository without .pom
files but only jars.
To do so, you can configure metadata sources for any repository.
repositories {
maven {
url = uri("http://repo.mycompany.com/repo")
metadataSources {
mavenPom()
artifact()
}
}
}
repositories {
maven {
url "http://repo.mycompany.com/repo"
metadataSources {
mavenPom()
artifact()
}
}
}
You can specify multiple sources to tell Gradle to keep looking if a file was not found. In that case, the order of checking for sources is predefined.
The following metadata sources are supported:
Metadata source | Description | Order | Maven | Ivy / flat dir |
---|---|---|---|---|
|
Look for Gradle |
1st |
yes |
yes |
|
Look for Maven |
2nd |
yes |
yes |
|
Look for |
2nd |
no |
yes |
|
Look directly for artifact |
3rd |
yes |
yes |
The defaults for Ivy and Maven repositories change with Gradle 6.0.
Before 6.0, artifact()
was included in the defaults.
Leading to some inefficiency when modules are missing completely.
To restore this behavior, for example, for Maven central you can use:
mavenCentral { metadataSources { mavenPom(); artifact() } }
In a similar way, you can opt into the new behavior in older Gradle versions using:
mavenCentral { metadataSources { mavenPom() } }
Since Gradle 5.3, when parsing a metadata file, be it Ivy or Maven, Gradle will look for a marker indicating that a matching Gradle Module Metadata files exists. If it is found, it will be used instead of the Ivy or Maven file.
Starting with Gradle 5.6, you can disable this behavior by adding ignoreGradleMetadataRedirection()
to the metadataSources declaration.
repositories {
maven {
url = uri("http://repo.mycompany.com/repo")
metadataSources {
mavenPom()
artifact()
ignoreGradleMetadataRedirection()
}
}
}
repositories {
maven {
url "http://repo.mycompany.com/repo"
metadataSources {
mavenPom()
artifact()
ignoreGradleMetadataRedirection()
}
}
}
Plugin repositories vs. build repositories
Gradle will use repositories at two different phases during your build.
The first phase is when configuring your build and loading the plugins it applied. To do that Gradle will use a special set of repositories.
The second phase is during dependency resolution. At this point Gradle will use the repositories declared in your project, as shown in the previous sections.
Plugin repositories
By default Gradle will use the Gradle plugin portal to look for plugins.
However, for different reasons, there are plugins available in other, public or not, repositories. When a build requires one of these plugins, additional repositories need to be specified so that Gradle knows where to search.
As the way to declare the repositories and what they are expected to contain depends on the way the plugin is applied, it is best to refer to Custom Plugin Repositories.
Centralizing repositories declaration
Instead of declaring repositories in every subproject of your build or via an allprojects
block, Gradle offers a way to declare them in a central place for all projects.
Central declaration of repositories is an incubating feature. |
Repositories used by convention in every subproject can be declared in the settings.gradle(.kts)
file:
dependencyResolutionManagement {
repositories {
mavenCentral()
}
}
dependencyResolutionManagement {
repositories {
mavenCentral()
}
}
The dependencyResolutionManagement
repositories block accepts the same notations as in a project. This includes Maven or Ivy repositories, with or without credentials, etc.
By default, repositories declared by a project in build.gradle(.kts)
will override whatever is declared in settings.gradle(.kts)
:
dependencyResolutionManagement {
repositoriesMode = RepositoriesMode.PREFER_PROJECT
}
dependencyResolutionManagement {
repositoriesMode = RepositoriesMode.PREFER_PROJECT
}
There are three modes for dependency resolution management:
Mode | Description | Default? | Use-Case |
---|---|---|---|
|
Any repository declared on a project will cause the project to use the repositories declared by the project, ignoring those declared in settings. |
Yes |
Useful when teams need to use different repositories not common among subprojects. |
|
Any repository declared directly in a project, either directly or via a plugin, will be ignored. |
No |
Useful for enforcing large teams to use approved repositories only, but will not fail the build when a project or plugin declares a repository. |
|
Any repository declared directly in a project, either directly or via a plugin, will trigger a build error. |
No |
Useful for enforcing large teams to use approved repositories only. |
You can change the behavior to prefer the repositories in the settings.gradle(.kts)
file by using repositoriesMode
:
dependencyResolutionManagement {
repositoriesMode = RepositoriesMode.PREFER_SETTINGS
}
dependencyResolutionManagement {
repositoriesMode = RepositoriesMode.PREFER_SETTINGS
}
Gradle will warn you if a project or a plugin declares a repository in a project.
You can force Gradle to fail the build if you want to enforce that only settings repositories are used:
dependencyResolutionManagement {
repositoriesMode = RepositoriesMode.FAIL_ON_PROJECT_REPOS
}
dependencyResolutionManagement {
repositoriesMode = RepositoriesMode.FAIL_ON_PROJECT_REPOS
}
Supported repository transport protocols
Maven and Ivy repositories support the use of various transport protocols. At the moment the following protocols are supported:
Type | Credential types | Link |
---|---|---|
|
none |
|
|
username/password |
|
|
username/password |
|
|
username/password |
|
|
access key/secret key/session token or Environment variables |
|
|
default application credentials sourced from well known files, Environment variables etc. |
Username and password should never be checked in plain text into version control as part of your build file.
You can store the credentials in a local gradle.properties file and use one of the open source Gradle plugins for encrypting and consuming credentials e.g. the credentials plugin.
|
The transport protocol is part of the URL definition for a repository. The following build script demonstrates how to create HTTP-based Maven and Ivy repositories:
repositories {
maven {
url = uri("http://repo.mycompany.com/maven2")
}
ivy {
url = uri("http://repo.mycompany.com/repo")
}
}
repositories {
maven {
url "http://repo.mycompany.com/maven2"
}
ivy {
url "http://repo.mycompany.com/repo"
}
}
The following example shows how to declare SFTP repositories:
repositories {
maven {
url = uri("sftp://repo.mycompany.com:22/maven2")
credentials {
username = "user"
password = "password"
}
}
ivy {
url = uri("sftp://repo.mycompany.com:22/repo")
credentials {
username = "user"
password = "password"
}
}
}
repositories {
maven {
url "sftp://repo.mycompany.com:22/maven2"
credentials {
username "user"
password "password"
}
}
ivy {
url "sftp://repo.mycompany.com:22/repo"
credentials {
username "user"
password "password"
}
}
}
For details on HTTP related authentication, see the section HTTP(S) authentication schemes configuration.
When using an AWS S3 backed repository you need to authenticate using AwsCredentials, providing access-key and a private-key. The following example shows how to declare a S3 backed repository and providing AWS credentials:
repositories {
maven {
url = uri("s3://myCompanyBucket/maven2")
credentials(AwsCredentials::class) {
accessKey = "someKey"
secretKey = "someSecret"
// optional
sessionToken = "someSTSToken"
}
}
ivy {
url = uri("s3://myCompanyBucket/ivyrepo")
credentials(AwsCredentials::class) {
accessKey = "someKey"
secretKey = "someSecret"
// optional
sessionToken = "someSTSToken"
}
}
}
repositories {
maven {
url "s3://myCompanyBucket/maven2"
credentials(AwsCredentials) {
accessKey "someKey"
secretKey "someSecret"
// optional
sessionToken "someSTSToken"
}
}
ivy {
url "s3://myCompanyBucket/ivyrepo"
credentials(AwsCredentials) {
accessKey "someKey"
secretKey "someSecret"
// optional
sessionToken "someSTSToken"
}
}
}
You can also delegate all credentials to the AWS sdk by using the AwsImAuthentication. The following example shows how:
repositories {
maven {
url = uri("s3://myCompanyBucket/maven2")
authentication {
create<AwsImAuthentication>("awsIm") // load from EC2 role or env var
}
}
ivy {
url = uri("s3://myCompanyBucket/ivyrepo")
authentication {
create<AwsImAuthentication>("awsIm")
}
}
}
repositories {
maven {
url "s3://myCompanyBucket/maven2"
authentication {
awsIm(AwsImAuthentication) // load from EC2 role or env var
}
}
ivy {
url "s3://myCompanyBucket/ivyrepo"
authentication {
awsIm(AwsImAuthentication)
}
}
}
For details on AWS S3 related authentication, see the section AWS S3 repositories configuration.
When using a Google Cloud Storage backed repository default application credentials will be used with no further configuration required:
repositories {
maven {
url = uri("gcs://myCompanyBucket/maven2")
}
ivy {
url = uri("gcs://myCompanyBucket/ivyrepo")
}
}
repositories {
maven {
url "gcs://myCompanyBucket/maven2"
}
ivy {
url "gcs://myCompanyBucket/ivyrepo"
}
}
For details on Google GCS related authentication, see the section Google Cloud Storage repositories configuration.
HTTP(S) authentication schemes configuration
When configuring a repository using HTTP or HTTPS transport protocols, multiple authentication schemes are available. By default, Gradle will attempt to use all schemes that are supported by the Apache HttpClient library, documented here. In some cases, it may be preferable to explicitly specify which authentication schemes should be used when exchanging credentials with a remote server. When explicitly declared, only those schemes are used when authenticating to a remote repository.
You can specify credentials for Maven repositories secured by basic authentication using PasswordCredentials.
repositories {
maven {
url = uri("http://repo.mycompany.com/maven2")
credentials {
username = "user"
password = "password"
}
}
}
repositories {
maven {
url "http://repo.mycompany.com/maven2"
credentials {
username "user"
password "password"
}
}
}
The following example show how to configure a repository to use only DigestAuthentication:
repositories {
maven {
url = uri("https://repo.mycompany.com/maven2")
credentials {
username = "user"
password = "password"
}
authentication {
create<DigestAuthentication>("digest")
}
}
}
repositories {
maven {
url 'https://repo.mycompany.com/maven2'
credentials {
username "user"
password "password"
}
authentication {
digest(DigestAuthentication)
}
}
}
Currently supported authentication schemes are:
- BasicAuthentication
-
Basic access authentication over HTTP. When using this scheme, credentials are sent preemptively.
- DigestAuthentication
-
Digest access authentication over HTTP.
- HttpHeaderAuthentication
-
Authentication based on any custom HTTP header, e.g. private tokens, OAuth tokens, etc.
Using preemptive authentication
Gradle’s default behavior is to only submit credentials when a server responds with an authentication challenge in the form of an HTTP 401 response. In some cases, the server will respond with a different code (ex. for repositories hosted on GitHub a 404 is returned) causing dependency resolution to fail. To get around this behavior, credentials may be sent to the server preemptively. To enable preemptive authentication simply configure your repository to explicitly use the BasicAuthentication scheme:
repositories {
maven {
url = uri("https://repo.mycompany.com/maven2")
credentials {
username = "user"
password = "password"
}
authentication {
create<BasicAuthentication>("basic")
}
}
}
repositories {
maven {
url 'https://repo.mycompany.com/maven2'
credentials {
username "user"
password "password"
}
authentication {
basic(BasicAuthentication)
}
}
}
Using HTTP header authentication
You can specify any HTTP header for secured Maven repositories requiring token, OAuth2 or other HTTP header based authentication using HttpHeaderCredentials with HttpHeaderAuthentication.
repositories {
maven {
url = uri("http://repo.mycompany.com/maven2")
credentials(HttpHeaderCredentials::class) {
name = "Private-Token"
value = "TOKEN"
}
authentication {
create<HttpHeaderAuthentication>("header")
}
}
}
repositories {
maven {
url "http://repo.mycompany.com/maven2"
credentials(HttpHeaderCredentials) {
name = "Private-Token"
value = "TOKEN"
}
authentication {
header(HttpHeaderAuthentication)
}
}
}
AWS S3 repositories configuration
S3 configuration properties
The following system properties can be used to configure the interactions with s3 repositories:
org.gradle.s3.endpoint
-
Used to override the AWS S3 endpoint when using a non AWS, S3 API compatible, storage service.
org.gradle.s3.maxErrorRetry
-
Specifies the maximum number of times to retry a request in the event that the S3 server responds with a HTTP 5xx status code. When not specified a default value of 3 is used.
S3 URL formats
S3 URL’s are 'virtual-hosted-style' and must be in the following format
s3://<bucketName>[.<regionSpecificEndpoint>]/<s3Key>
e.g. s3://myBucket.s3.eu-central-1.amazonaws.com/maven/release
-
myBucket
is the AWS S3 bucket name. -
s3.eu-central-1.amazonaws.com
is the optional region specific endpoint. -
/maven/release
is the AWS S3 key (unique identifier for an object within a bucket)
S3 proxy settings
A proxy for S3 can be configured using the following system properties:
-
https.proxyHost
-
https.proxyPort
-
https.proxyUser
-
https.proxyPassword
-
http.nonProxyHosts
(NOTE: this is not a typo.)
If the org.gradle.s3.endpoint
property has been specified with a HTTP (not HTTPS) URI the following system proxy settings can be used:
-
http.proxyHost
-
http.proxyPort
-
http.proxyUser
-
http.proxyPassword
-
http.nonProxyHosts
AWS S3 V4 Signatures (AWS4-HMAC-SHA256)
Some of the AWS S3 regions (eu-central-1 - Frankfurt) require that all HTTP requests are signed in accordance with AWS’s signature version 4. It is recommended to specify S3 URL’s containing the region specific endpoint when using buckets that require V4 signatures. e.g.
s3://somebucket.s3.eu-central-1.amazonaws.com/maven/release
When a region-specific endpoint is not specified for buckets requiring V4 Signatures, Gradle will use the default AWS region (us-east-1) and the following warning will appear on the console:
Attempting to re-send the request to .... with AWS V4 authentication. To avoid this warning in the future, use region-specific endpoint to access buckets located in regions that require V4 signing.
Failing to specify the region-specific endpoint for buckets requiring V4 signatures means:
-
3 round-trips to AWS, as opposed to one, for every file upload and download.
-
Depending on location - increased network latencies and slower builds.
-
Increased likelihood of transmission failures.
AWS S3 Cross Account Access
Some organizations may have multiple AWS accounts, e.g. one for each team. The AWS account of the bucket owner is often different from the artifact publisher and consumers. The bucket owner needs to be able to grant the consumers access otherwise the artifacts will only be usable by the publisher’s account. This is done by adding the bucket-owner-full-control
Canned ACL to the uploaded objects. Gradle will do this in every upload. Make sure the publisher has the required IAM permission, PutObjectAcl
(and PutObjectVersionAcl
if bucket versioning is enabled), either directly or via an assumed IAM Role (depending on your case). You can read more at AWS S3 Access Permissions.
Google Cloud Storage repositories configuration
GCS configuration properties
The following system properties can be used to configure the interactions with Google Cloud Storage repositories:
org.gradle.gcs.endpoint
-
Used to override the Google Cloud Storage endpoint when using a non-Google Cloud Platform, Google Cloud Storage API compatible, storage service.
org.gradle.gcs.servicePath
-
Used to override the Google Cloud Storage root service path which the Google Cloud Storage client builds requests from, defaults to
/
.
GCS URL formats
Google Cloud Storage URL’s are 'virtual-hosted-style' and must be in the following format gcs://<bucketName>/<objectKey>
e.g. gcs://myBucket/maven/release
-
myBucket
is the Google Cloud Storage bucket name. -
/maven/release
is the Google Cloud Storage key (unique identifier for an object within a bucket)
Handling credentials
Repository credentials should never be part of your build script but rather be kept external. Gradle provides an API in artifact repositories that allows you to declare only the type of required credentials. Credential values are looked up from the Gradle Properties during the build that requires them.
For example, given repository configuration:
repositories {
maven {
name = "mySecureRepository"
credentials(PasswordCredentials::class)
// url = uri(<<some repository url>>)
}
}
repositories {
maven {
name = 'mySecureRepository'
credentials(PasswordCredentials)
// url = uri(<<some repository url>>)
}
}
The username and password will be looked up from mySecureRepositoryUsername
and mySecureRepositoryPassword
properties.
Note that the configuration property prefix - the identity - is determined from the repository name.
Credentials can then be provided in any of supported ways for Gradle Properties -
gradle.properties
file, command line arguments, environment variables or a combination of those options.
Also, note that credentials will only be required if the invoked build requires them. If for example a project is configured to publish artifacts to a secured repository, but the build does not invoke publishing task, Gradle will not require publishing credentials to be present. On the other hand, if the build needs to execute a task that requires credentials at some point, Gradle will check for credential presence first thing and will not start running any of the tasks if it knows that the build will fail at a later point because of missing credentials.
Here is a downloadable sample that demonstrates the concept in more detail.
Lookup is only supported for credentials listed in the Table 3.
Type | Argument | Base property name | Required? |
---|---|---|---|
|
|
required |
|
|
|
required |
|
|
|
required |
|
|
|
required |
|
|
|
optional |
|
|
|
required |
|
|
|
required |