Part 2: The Build Lifecycle
Learn about the Gradle build lifecycle and what each phase represents.
Step 0. Before you Begin
-
You initialized your Java app in part 1.
Step 1. Understanding the Build Lifecycle
A Gradle build has three distinct phases:
- Phase 1 - Initialization
-
During the initialization phase, Gradle determines which projects will take part in the build, and creates a
Project
instance for each project. - Phase 2 - Configuration
-
During the configuration phase, the
Project
objects are configured using the build scripts of all projects in the build. Gradle determines the set of tasks to be executed. - Phase 3 - Execution
-
During the execution phase, Gradle executes each of the selected tasks.
When Gradle is invoked to execute a task, the lifecycle begins. Let’s see it in action.
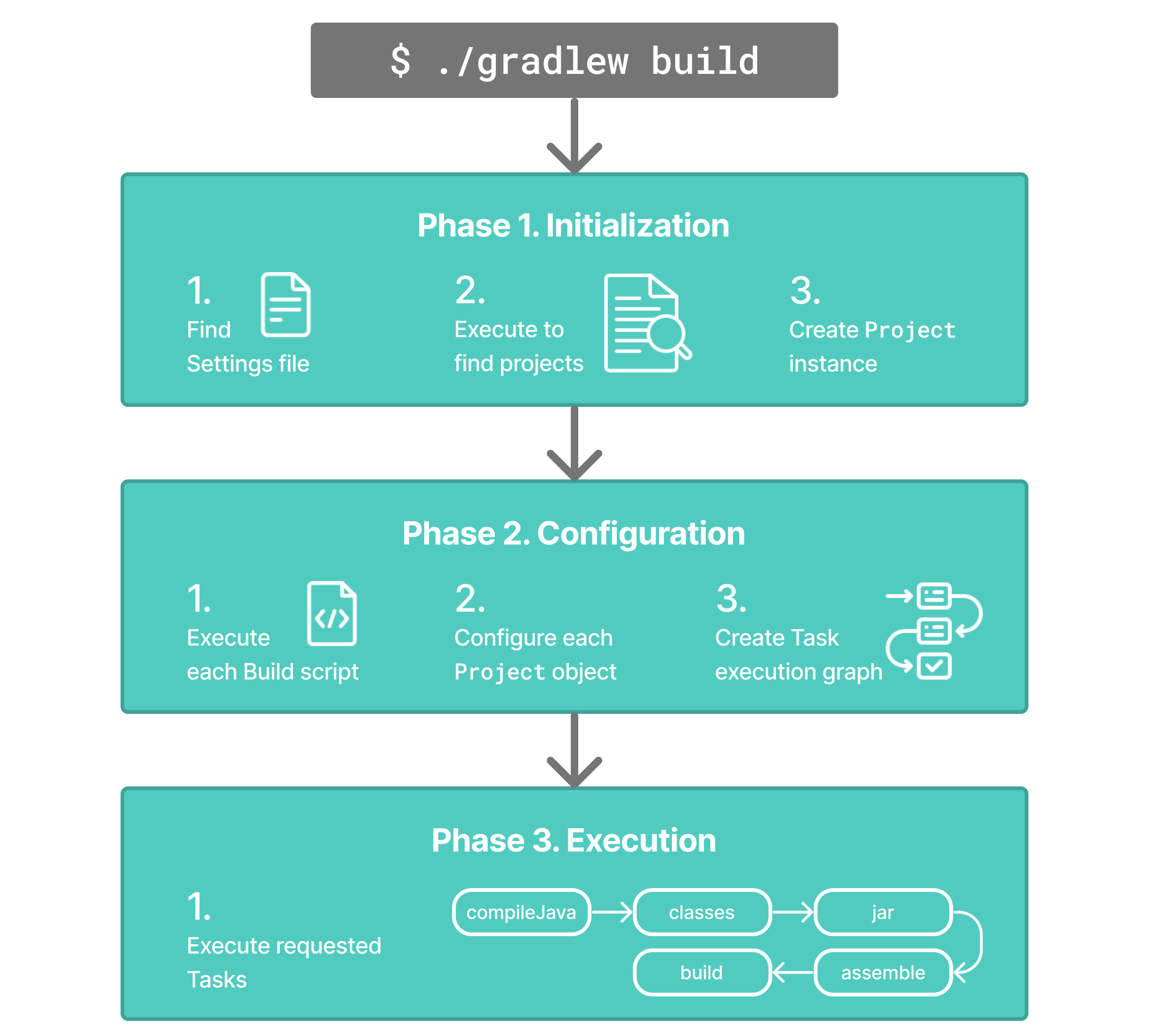
Step 2. Update the Settings File
Add the following line to the top of the Settings file:
println("SETTINGS FILE: This is executed during the initialization phase")
println('SETTINGS FILE: This is executed during the initialization phase')
Step 3. Update the Build Script
Add the following lines to the bottom of the Build script:
println("BUILD SCRIPT: This is executed during the configuration phase")
tasks.register("task1"){
println("REGISTER TASK1: This is executed during the configuration phase")
}
tasks.register("task2"){
println("REGISTER TASK2: This is executed during the configuration phase")
}
tasks.named("task1"){
println("NAMED TASK1: This is executed during the configuration phase")
doFirst {
println("NAMED TASK1 - doFirst: This is executed during the execution phase")
}
doLast {
println("NAMED TASK1 - doLast: This is executed during the execution phase")
}
}
tasks.named("task2"){
println("NAMED TASK2: This is executed during the configuration phase")
doFirst {
println("NAMED TASK2 - doFirst: This is executed during the execution phase")
}
doLast {
println("NAMED TASK2 - doLast: This is executed during the execution phase")
}
}
println("BUILD SCRIPT: This is executed during the configuration phase")
tasks.register("task1") {
println("REGISTER TASK1: This is executed during the configuration phase")
}
tasks.register("task2") {
println("REGISTER TASK2: This is executed during the configuration phase")
}
tasks.named("task1") {
println("NAMED TASK1: This is executed during the configuration phase")
doFirst {
println("NAMED TASK1 - doFirst: This is executed during the execution phase")
}
doLast {
println("NAMED TASK1 - doLast: This is executed during the execution phase")
}
}
tasks.named("task2") {
println("NAMED TASK2: This is executed during the configuration phase")
doFirst {
println("NAMED TASK2 - doFirst: This is executed during the execution phase")
}
doLast {
println("NAMED TASK2 - doLast: This is executed during the execution phase")
}
}
Step 4. Run a Gradle Task
Run the task1
task that you registered and configured in Step 3:
$ ./gradlew task1
SETTINGS FILE: This is executed during the initialization phase (1)
> Configure project :app
BUILD SCRIPT: This is executed during the configuration phase (2)
REGISTER TASK1: This is executed during the configuration phase (2)
NAMED TASK1: This is executed during the configuration phase (2)
> Task :app:task1
NAMED TASK1 - doFirst: This is executed during the execution phase (3)
NAMED TASK1 - doLast: This is executed during the execution phase (3)
BUILD SUCCESSFUL in 25s
5 actionable tasks: 3 executed, 2 up-to-date
1 | Initialization: Gradle executes settings.gradle(.kts) to determine the projects to be built and creates a Project object for each one. |
2 | Configuration: Gradle configures each project by executing the build.gradle(.kts) files. It resolves dependencies and creates a dependency graph of all the available tasks. |
3 | Execution: Gradle executes the tasks passed on the command line and any prerequisite tasks. |
It is important to note that while task1
was configured and executed, task2
was not.
This is called task configuration avoidance and prevents unnecessary work.
Task configuration avoidance is when Gradle avoids configuring task2
when task1
was called and task1
does NOT depend. on task2
.
Next Step: Multi-Project Builds >>