Declaring Repositories Basics
Gradle can resolve local or external dependencies from one or many repositories based on Maven, Ivy or flat directory formats.
Repositories intended for use in a single project are declared in your build.gradle(.kts)
file:
Kotlin
Groovy
repositories {
mavenCentral()
maven {
url = 'https://repo.spring.io/snapshot/'
}
}
To centralize repository declarations in your settings.gradle(.kts)
file, head over to Centralizing Repository Declarations.
Declaring a publicly-available repository
Organizations building software may want to leverage public binary repositories to download and consume publicly available dependencies. Popular public repositories include Maven Central and the Google Android repository.
Gradle provides built-in shorthand notations for these widely-used repositories.
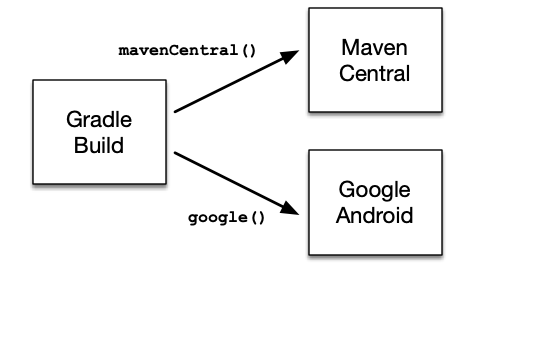
Under the covers, Gradle resolves dependencies from the respective URL of the public repository defined by the shorthand notation. All shorthand notations are available via the RepositoryHandler API.
Alternatively, you can explicitly specify the URL of the repository for more fine-grained control.
Maven Central repository
Maven Central is a popular repository hosting open source libraries for consumption by Java projects.
To declare the Maven Central repository for your build add this to your script:
Kotlin
Groovy
repositories {
mavenCentral()
}
Google Maven repository
The Google repository hosts Android-specific artifacts including the Android SDK. For usage examples, see the relevant Android documentation.
To declare the Google Maven repository add this to your build script:
Kotlin
Groovy
repositories {
google()
}
Declaring a custom repository by URL
Most enterprise projects set up a binary repository available only within an intranet. In-house repositories enable teams to publish internal binaries, setup user management and security measures, and ensure uptime and availability.
Specifying a custom URL is also helpful if you want to declare publicly-available repository that Gradle does not provide a shorthand for.
Repositories with custom URLs can be specified as Maven or Ivy repositories by calling the corresponding methods available on the RepositoryHandler API:
Kotlin
Groovy
repositories {
maven {
url = "http://repo.mycompany.com/maven2"
}
}
Gradle supports additional protocols beyond http
and https
, such as file
, sftp
, and s3
for custom URLs.
For full coverage, see the section on supported repository types.
You can also define your own repository layout by using ivy { }
repositories, as they are very flexible in terms of how modules are organised in a repository:
Kotlin
Groovy
repositories {
ivy {
url = "http://repo.mycompany.com/repo"
}
}
Declaring multiple repositories
You can define more than one repository for resolving dependencies. Declaring multiple repositories is helpful if some dependencies are only available in one repository but not the other.
You can mix any type of repository described in the reference section.
Kotlin
Groovy
repositories {
mavenCentral()
maven {
url = "https://repo.spring.io/release"
}
maven {
url = "https://repository.jboss.org/maven2"
}
}
The order of repository declaration determines the order that Gradle will search for dependencies during resolution. If Gradle finds a dependency’s metadata in a particular repository, it will attempt to download all the artifacts for that module from the same repository.
You can learn more about the inner workings of dependency downloads.
Plugin repositories
Gradle uses a different set of repositories for resolving Gradle plugins and resolving project dependencies:
-
Plugin dependencies: When resolving plugins for build scripts, Gradle uses a distinct set of repositories to locate and load the required plugins.
-
Project dependencies: When resolving project dependencies, Gradle only uses the repositories declared in the build script and ignores the plugin repositories.
By default, Gradle uses the Gradle Plugin Portal to search for plugins:
Kotlin
Groovy
pluginManagement {
repositories {
mavenCentral()
gradlePluginPortal()
}
}
However, some plugins may be hosted in other repositories (public or private). To include these plugins, you need to specify additional repositories in your build script so Gradle knows where to search.
Since declaring repositories depends on how the plugin is applied, refer to the Custom Plugin Repositories for more details on configuring repositories for plugins from different sources.