The C++ Unit Test Plugin provides the tasks, configurations and conventions for integrating with a C++ executable-based testing framework, such as Google Test.
Usage
Kotlin
Groovy
plugins {
id 'cpp-unit-test'
}
Build variants
The C++ Unit Test Plugin understands the following dimensions. Read the introduction to build variants for more information.
- Target machines - defaults to the tested component (if present) or build host (otherwise)
-
The target machine expresses which machines the application expects to run. A target machine is identified by its operating system and architecture. Gradle uses the target machine to decide which tool chain to choose based on availability on the host machine.
The target machine can be configured as follows:
Kotlin
Groovy
unitTest {
targetMachines = [
machines.linux.x86_64,
machines.windows.x86, machines.windows.x86_64,
machines.macOS.x86_64
]
}
Tasks
The following diagram shows the relationships between tasks added by this plugin.

Variant-dependent Tasks
The C++ Unit Test Plugin creates tasks based on the variants of the unit test component. Read the introduction to build variants for more information. The following diagrams show the relationship between variant-dependent tasks.

compileTestVariantCpp
(e.g.compileTestCpp
) - CppCompile-
Depends on: All tasks that contribute source files to the compilation :: Compiles C++ source files using the selected compiler.
linkTestVariant
(e.g.linkTest
) - LinkExecutable-
Depends on: All tasks which contribute to the link executable, including
linkVariant
andcreateVariant
tasks from projects that are resolved via project dependencies and tested component :: Links executable from compiled object files using the selected linker. installTestVariant
(e.g.installTest
) - InstallExecutable-
Depends on:
linkTestVariant
and all tasks which contribute to the runtime of the executable, includinglinkVariant
tasks from projects that are resolved via project dependencies :: Installs the executable and all of it’s runtime dependencies for easy execution. runTestVariant
(e.g.runTest
) - RunTestExecutable-
Depends on:
installTestVariant
:: Run the installed executable.
Lifecycle Tasks
The C++ Unit Test Plugin attaches some of its tasks to the standard lifecycle tasks documented in the Base Plugin chapter — which the C++ Unit Test Plugin applies automatically:
assemble
- Task (lifecycle)-
Aggregate task that assembles the debug variant of the tested component for the current host (if present) in the project. For example, the C++ Application Plugin and C++ Library Plugin attach their link and create tasks to this lifecycle task. This task is added by the Base Plugin.
test
- Task (lifecycle)-
Depends on:
runTestVariant
that most closely matches the build host :: Aggregate task of the variant that most closely match the build host for testing the component. check
- Task (lifecycle)-
Depends on:
test
:: Aggregate task that performs verification tasks, such as running the tests. Some plugins add their own verification task tocheck
. This task is added by the Base Plugin. build
- Task (lifecycle)-
Depends on:
check
,assemble
:: Aggregate tasks that perform a full build of the project. This task is added by the Base Plugin. clean
- Delete-
Deletes the build directory and everything in it, i.e. the path specified by the
layout.buildDirectory
project property. This task is added by the Base Plugin.
Dependency management
Just like the tasks created by the C++ Unit Test Plugin, the configurations are created based on the variant of the application component. Read the introduction to build variants for more information. The following graph describes the configurations added by the C++ Unit Test Plugin:
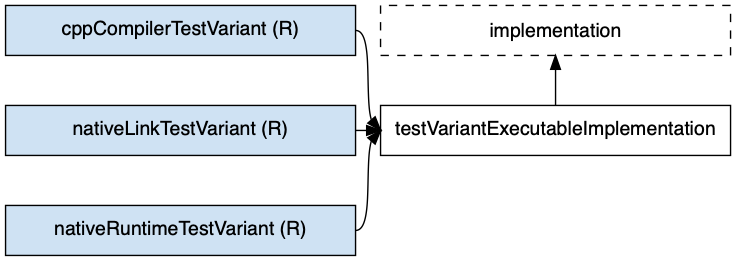
-
The configurations in white are the ones a user should use to declare dependencies
-
The configurations in blue, also known as resolvable denoted by (R), are internal to the component, for its own use
The following configurations are used to declare dependencies:
testImplementation
-
Used for declaring implementation dependencies for all variants of the test component. This is where you should declare dependencies of any variants. Note this configuration inherit all dependencies declared on the tested component (library or application).
testVariantExecutableImplementation
(e.g.testExecutableImplementation
) extendstestImplementation
-
Used for declaring implementation dependencies for a specific variant of the test component. This is where you should declare dependencies of the specific variant.
There is no configurations that can be used by consumers for this plugin.
The following configurations are used by the unit test itself:
cppCompileTestVariant
(e.g.cppCompileTest
) extendstestVariantExecutableImplementation
-
Used for compiling the unit test. This configuration contains the compile include roots of the unit test and is therefore used when invoking the C++ compiler to compile it.
nativeLinkTestVariant
(e.g.nativeLinkTest
) extendstestVariantExecutableImplementation
-
Used for linking the unit test. This configuration contains the libraries of the unit test and is therefore used when invoking the C++ linker to link it.
nativeRuntimeTestVariant
(e.g.nativeRuntimeTest
) extendstestVariantExecutableImplementation
-
Used for executing the unit test. This configuration contains the runtime libraries of the unit test.
Conventions
The C++ Unit Test Plugin adds conventions for sources and tasks, shown below.
Project layout
The C++ Unit Test Plugin assumes the project layout shown below. None of these directories needs to exist or have anything in them. The C++ Unit Test Plugin will compile whatever it finds, and handles anything which is missing.
src/test/cpp
-
C++ source with extension of
.cpp
,.c++
or.cc
src/test/headers
-
Headers - headers needed to compile the unit test
You configure the project layout by configuring the source
and privateHeaders
respectively on the unitTest
script block.
compileTestVariantCpp
Task
The C++ Unit Test Plugin adds a CppCompile instance for each variant of the test component to build (e.g. compileTestCpp
).
Read the introduction to build variants for more information.
Some of the most common configuration options are shown below.
compilerArgs |
[] |
debuggable |
|
includes |
|
macros |
[:] |
objectFileDir |
|
optimized |
|
positionIndependentCode |
|
source |
|
systemIncludes |
derived from the tool chain |
targetPlatform |
derived from the |
toolChain |
linkTestVariant
Task
The C++ Unit Test Plugin adds a LinkExecutable instance for each variant of the test component - e.g. linkTest
.
Read the introduction to build variants for more information.
Some of the most common configuration options are shown below.
debuggable |
|
libs |
|
linkedFile |
|
linkerArgs |
[] |
source |
|
targetPlatform |
derived from the |
toolChain |
installTestVariant
Task
The C++ Unit Test Plugin adds a InstallExecutable instance for each variant of the test component - e.g. installTest
.
Read the introduction to build variants for more information.
There is no need to configure any properties on the task.
runTestVariant
Task
The C++ Unit Test Plugin adds a RunTestExecutable instance for each variant of the test component - e.g. runTest
.
Read the introduction to build variants for more information.
Some of the most common configuration options are shown below.
args |
|
ignoreFailures |
|