The Swift Library Plugin provides the tasks, conventions and conventions for building a Swift library. In particular, a Swift library provides functionality that can be used by consumers (i.e., other projects using this plugin or the Swift Application Plugin).
Usage
plugins {
`swift-library`
}
plugins {
id 'swift-library'
}
Build variants
The Swift Library Plugin understands the following dimensions. Read the introduction to build variants for more information.
- Build types - always set to debug and release
-
The build type controls the debuggability as well as the optimization of the generated binaries.
-
debug
- Generate debug symbols and don’t optimized the binary -
release
- Generate debug symbols and optimize, but extract the debug symbols from the binary
-
- Linkages - default to shared
-
The linkage expresses whether a shared library or static library should be created. Libraries can produce a shared library, a static library or both.
The linkage can be configured as follows:
library {
linkage = listOf(Linkage.STATIC, Linkage.SHARED)
}
library {
linkage = [Linkage.STATIC, Linkage.SHARED]
}
- Target machines - defaults to the build host
-
The target machine expresses which machines the application expects to run. A target machine is identified by its operating system and architecture. Gradle uses the target machine to decide which tool chain to choose based on availability on the host machine.
The target machine can be configured as follows:
library {
targetMachines = listOf(machines.linux.x86_64, machines.macOS.x86_64)
}
library {
targetMachines = [
machines.linux.x86_64,
machines.macOS.x86_64
]
}
Tasks
The following diagram shows the relationships between tasks added by this plugin.
Note the default linkage of a Swift library is shared linkage as shown in the diagram.

With static linkage, the diagram changes to the following:

Variant-dependent Tasks
The Swift Library Plugin creates tasks based on variants of the library component. Read the introduction to build variants for more information. The following diagrams show the relationship between variant-dependent tasks.

Depending on the linkage property |
compileVariantSwift
(e.g.compileDebugSwift
andcompileReleaseSwift
) - SwiftCompile-
Depends on: All tasks that contribute source files to the compilation :: Compiles Swift source files using the selected compiler.
linkVariant
(e.g.linkDebug
andlinkRelease
) - LinkSharedLibrary (shared linkage)-
Depends on: All tasks which contribute to the link libraries, including
linkVariant
andcreateVariant
tasks from projects that are resolved via project dependencies :: Links shared library from compiled object files using the selected linker. createVariant
(e.g.createDebug
andcreateRelease
) - CreateStaticLibrary (static linkage)-
Creates static library from compiled object files using selected archiver
assembleVariant
(e.g.assembleDebug
andassembleRelease
) - Task (lifecycle)-
Depends on:
linkVariant
(shared linkage) orcreateVariant
(static linkage) :: Aggregates tasks that assemble the specific variant of this library.
Lifecycle Tasks
The Swift Library Plugin attaches some of its tasks to the standard lifecycle tasks documented in the Base Plugin chapter — which the Swift Library Plugin applies automatically:
assemble
- Task (lifecycle)-
Depends on:
linkDebug
when linkage includesshared
orcreateDebug
otherwise. :: Aggregate task that assembles the debug variant of the shared library (if available) for the current host (if present) in the project. This task is added by the Base Plugin. check
- Task (lifecycle)-
Aggregate task that performs verification tasks, such as running the tests. Some plugins add their own verification task to
check
. For example, the XCTest Plugin attach its test task to this lifecycle task. This task is added by the Base Plugin. build
- Task (lifecycle)-
Depends on:
check
,assemble
:: Aggregate tasks that perform a full build of the project. This task is added by the Base Plugin. clean
- Delete-
Deletes the build directory and everything in it, i.e. the path specified by the
layout.buildDirectory
project property. This task is added by the Base Plugin.
Dependency management
Just like the tasks created by the Swift Library Plugin, multiple configurations are created based on the variants of the library component. Read the introduction to build variants for more information. The following graph describes the configurations added by the Swift Library Plugin:
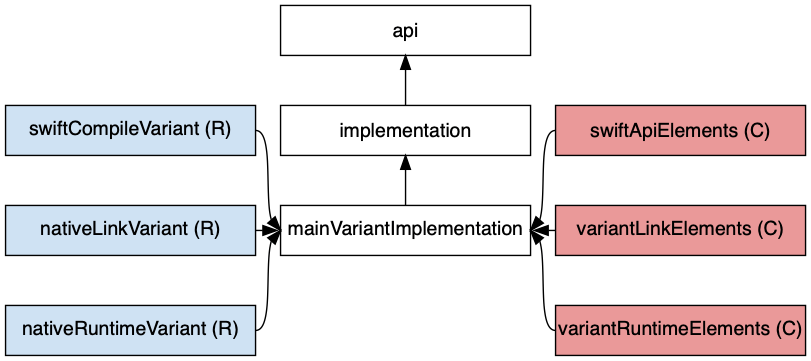
-
The configurations in white are the ones a user should use to declare dependencies
-
The configurations in pink, also known as consumable denoted by (C), are the ones used when a component compiles, links, or runs against the library
-
The configurations in blue, also known as resolvable denoted by (R), are internal to the component, for its own use
The following configurations are used to declare dependencies:
api
-
Used for declaring API dependencies (see API vs implementation section). This is where you should declare dependencies which are transitively exported to consumers, for compile and link.
implementation
extendsapi
-
Used for declaring implementation dependencies for all variants of the main component (see API vs implementation section). This is where you should declare dependencies which are purely internal and not meant to be exposed to consumers of any variants.
mainVariantImplementation
(e.g.mainDebugImplementation
andmainReleaseImplementation
) extendsimplementation
-
Used for declaring implementation dependencies for a specific variant of the main component (see API vs implementation section). This is where you should declare dependencies which are purely internal and not meant to be exposed to consumers of this specific variant.
The following configurations are used by consumers:
variantSwiftApiElements
(e.g.debugSwiftApiElements
andreleaseSwiftApiElements
) extendsmainVariantImplementation
-
Used for compiling against the library. This configuration is meant to be used by consumers, to retrieve all the elements necessary to compile against the library.
variantLinkElements
(e.g.debugLinkElements
andreleaseLinkElements
) extendsmainVariantImplementation
-
Used for linking against the library. This configuration is meant to be used by consumers, to retrieve all the elements necessary to link against the library.
variantRuntimeElements
(e.g.debugRuntimeElements
andreleaseRuntimeElements) extends `mainVariantImplementation
-
Used for executing the library. This configuration is meant to be used by consumers, to retrieve all the elements necessary to run against the library.
The following configurations are used by the library itself:
swiftCompileVariant
(e.g.swiftCompileDebug
andswiftCompileRelease
) extendsmainVariantImplementation
-
Used for compiling the library. This configuration contains the compile include roots of the library and is therefore used when invoking the Swift compiler to compile it.
nativeLinkVariant
(e.g.nativeLinkDebug
andnativeLinkRelease
) extendsmainVariantImplementation
-
Used for linking the library the shared library only. This configuration contains the libraries of the library and is therefore used when invoking the Swift linker to link it.
nativeRuntimeVariant
(e.g.nativeRuntimeDebug
andnativeRuntimeRelease
) extendsmainVariantImplementation
-
Used for executing the library. This configuration contains the runtime libraries of the library.
API vs implementation
The plugin exposes two configurations that can be used to declare dependencies: api
and implementation
.
The api
configuration should be used to declare dependencies which are exported by the library API, whereas the implementation
configuration should be used to declare dependencies which are internal to the component.
library {
dependencies {
// FIXME: Put real deps here.
api("io.qt:core:5.1")
implementation("io.qt:network:5.1")
}
}
library {
dependencies {
// FIXME: Write better deps here.
api "io.qt:core:5.1"
implementation "io.qt:network:5.1"
}
}
Dependencies appearing in the api
configurations will be transitively exposed to consumers of the library, and as such will appear on the compile include root and link libraries of consumers.
Dependencies found in the implementation
configuration will, on the other hand, not be exposed to consumers, and therefore not leak into the consumer’s compile include root and link libraries.
This comes with several benefits:
-
dependencies do not leak into the compile include roots and link libraries of consumers, so they can never accidentally depend on a transitive dependency
-
faster compilation thanks to the reduced include roots and link libraries
-
fewer recompilations when implementation dependencies change since the consumer would not need to be recompiled
Conventions
The Swift Library Plugin adds conventions for sources and tasks, shown below.
Project layout
The Swift Library Plugin assumes the project layout shown below. None of these directories needs to exist or have anything in them. The Swift Library Plugin will compile whatever it finds and ignore anything missing.
src/main/swift
-
Swift source with extension of
.swift
You configure the project layout by configuring the source
on the library
script block.
compileVariantSwift
Task
The Swift Library Plugin adds a SwiftCompile instance for each variant of the library component to build (e.g. compileDebugSwift
and compileReleaseSwift
).
Read the introduction to build variants for more information.
Some of the most common configuration options are shown below.
compilerArgs |
[] |
debuggable |
|
modules |
|
macros |
[] |
objectFileDir |
|
optimized |
|
source |
|
targetPlatform |
derived from the |
toolChain |
linkVariant
Task
The Swift Library Plugin adds a LinkSharedLibrary instance for each variant of the library containing shared linkage as a dimension - e.g. linkDebug
and linkRelease
.
Read the introduction to build variants for more information.
Some of the most common configuration options are shown below.
debuggable |
|
libs |
|
linkedFile |
|
linkerArgs |
[] |
source |
|
targetPlatform |
derived from the |
toolChain |
createVariant
Task
The Swift Library Plugin adds a CreateStaticLibrary instance for each variant of the library containing static linkage as a dimension - e.g. createDebug
and createRelease
.
Read the introduction to build variants for more information.
Some of the most common configuration options are shown below.
outputFile |
|
source |
|
staticLibArgs |
[] |
targetPlatform |
derived from the |
toolChain |