The XCTest Plugin is not compatible with the configuration cache. |
The XCTest Plugin provides the tasks, configurations and conventions for integrating with a XCTest testing framework on macOS as well as Linux’s open source implementation.
Usage
plugins {
xctest
}
plugins {
id 'xctest'
}
Build variants
The XCTest Plugin understands the following dimensions. Read the introduction to build variants for more information.
- Target machines - defaults to the tested component (if present) or build host (otherwise)
-
The target machine expresses which machines the application expects to run. A target machine is identified by its operating system and architecture. Gradle uses the target machine to decide which tool chain to choose based on availability on the host machine.
The target machine can be configured as follows:
xctest {
targetMachines = listOf(machines.linux.x86_64, machines.macOS.x86_64)
}
xctest {
targetMachines = [
machines.linux.x86_64,
machines.macOS.x86_64
]
}
Tasks
The following diagram shows the relationships between tasks added by this plugin.

Variant-dependent Tasks
The XCTest Plugin creates tasks based on the variants of the test component. Read the introduction to build variants for more information. The following diagrams show the relationship between variant-dependent tasks.

compileTestVariantSwift
(e.g.compileTestSwift
) - SwiftCompile-
Depends on: All tasks that contribute source files to the compilation :: Compiles Swift source files using the selected compiler.
linkTestVariant
(e.g.linkTest
) - LinkMachOBundle (on macOS) or LinkExecutable (on Linux)-
Depends on: All tasks which contribute to the link executable, including
linkVariant
andcreateVariant
tasks from projects that are resolved via project dependencies and tested component :: Links executable from compiled object files using the selected linker. installTestVariant
(e.g.installTest
) - InstallXCTestBundle (on macOS) or InstallExecutable (on Linux)-
Depends on:
linkTestVariant
and all tasks which contribute to the runtime of the executable, includinglinkVariant
tasks from projects that are resolved via project dependencies :: Installs the executable and all of it’s runtime dependencies for easy execution. xcTestVariant
(e.g.xcTest
) - XCTest-
Depends on:
installTestVariant
:: Run the installed executable.
Lifecycle Tasks
The XCTest Plugin attaches some of its tasks to the standard lifecycle tasks documented in the Base Plugin chapter — which the XCTest Plugin applies automatically:
assemble
- Task (lifecycle)-
Aggregate task that assembles the debug variant of the tested component for the current host (if present) in the project. For example, the Swift Application Plugin and Swift Library Plugin attach their link and create tasks to this lifecycle task. This task is added by the Base Plugin.
test
- Task (lifecycle)-
Depends on:
xcTestVariant
that most closely matches the build host :: Aggregate task of the variant that most closely match the build host for testing the component. check
- Task (lifecycle)-
Depends on:
test
:: Aggregate task that performs verification tasks, such as running the tests. Some plugins add their own verification task tocheck
. This task is added by the Base Plugin. build
- Task (lifecycle)-
Depends on:
check
,assemble
:: Aggregate tasks that perform a full build of the project. This task is added by the Base Plugin. clean
- Delete-
Deletes the build directory and everything in it, i.e. the path specified by the
layout.buildDirectory
project property. This task is added by the Base Plugin.
Dependency management
Just like the tasks created by the XCTest Plugin, the configurations are created based on the variant of the application component. Read the introduction to build variants for more information. The following graph describes the configurations added by the XCTest Plugin:
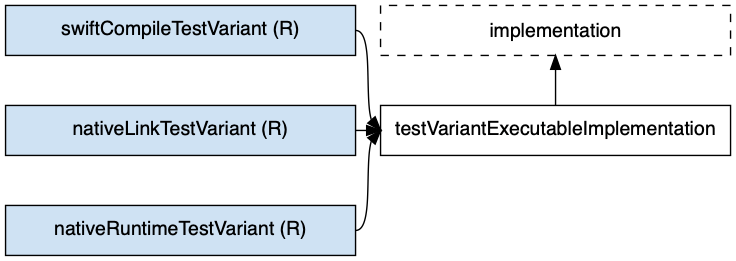
-
The configurations in white are the ones a user should use to declare dependencies
-
The configurations in blue, also known as resolvable denoted by (R), are internal to the component, for its own use
The following configurations are used to declare dependencies:
testImplementation
-
Used for declaring implementation dependencies for all variants of the test component. This is where you should declare dependencies of any variants. Note this configuration inherit all dependencies declared on the tested component (library or application).
testVariantExecutableImplementation
(e.g.testExecutableImplementation
) extendstestImplementation
-
Used for declaring implementation dependencies for a specific variant of the test component. This is where you should declare dependencies of the specific variant.
There is no configurations that can be used by consumers for this plugin.
The following configurations are used by the test component itself:
swiftCompileTestVariant
(e.g.swiftCompileTest
) extendstestVariantExecutableImplementation
-
Used for compiling the test component. This configuration contains the compile module of the test component and is therefore used when invoking the Swift compiler to compile it.
nativeLinkTestVariant
(e.g.nativeLinkTest
) extendstestVariantExecutableImplementation
-
Used for linking the test component. This configuration contains the libraries of the test component and is therefore used when invoking the Swift linker to link it.
nativeRuntimeTestVariant
(e.g.nativeRuntimeTest
) extendstestVariantExecutableImplementation
-
Used for executing the test component. This configuration contains the runtime libraries of the test component.
Conventions
The XCTest Plugin adds conventions for sources and tasks, shown below.
Project layout
The XCTest Plugin assumes the project layout shown below. None of these directories needs to exist or have anything in them. The XCTest Plugin will compile whatever it finds, and handles anything which is missing.
src/test/swift
-
Swift source with extension of
.swift
You configure the project layout by configuring the source
on the xctest
script block.
compileTestVariantSwift
Task
The XCTest Plugin adds a SwiftCompile instance for each variant of the test component to build (e.g. compileTestSwift
).
Read the introduction to build variants for more information.
Some of the most common configuration options are shown below.
compilerArgs |
[] |
debuggable |
|
modules |
|
macros |
[] |
objectFileDir |
|
optimized |
|
source |
|
targetPlatform |
derived from the |
toolChain |
linkTestVariant
Task
The XCTest Plugin adds a LinkMachOBundle instance on macOS or LinkExecutable instance on Linux for each variant of the test component - e.g. linkTest
.
Read the introduction to build variants for more information.
Some of the most common configuration options are shown below.
macOS
debuggable |
|
libs |
|
linkedFile |
|
linkerArgs |
[] |
source |
|
targetPlatform |
derived from the |
toolChain |
Linux
debuggable |
|
libs |
|
linkedFile |
|
linkerArgs |
[] |
source |
|
targetPlatform |
derived from the |
toolChain |
installTestVariant
Task
The XCTest Plugin adds a InstallXCTestBundle instance on macOS or InstallExecutable instance on Linux for each variant of the test component - e.g. installTest
.
Read the introduction to build variants for more information.
There is no need to configure any properties on the task.
xcTestVariant
Task
The XCTest Plugin adds a XCTest instance for each variant of the test component - e.g. xcTest
.
Read the introduction to build variants for more information.
Some of the most common configuration options are shown below.
binResultDir |
|
ignoreFailures |
|
reports |
|
runScriptFile |
|
testInstallDirectory |
|
workingDirectory |
|