Writing Settings Files
The settings file is the entry point of every Gradle build.
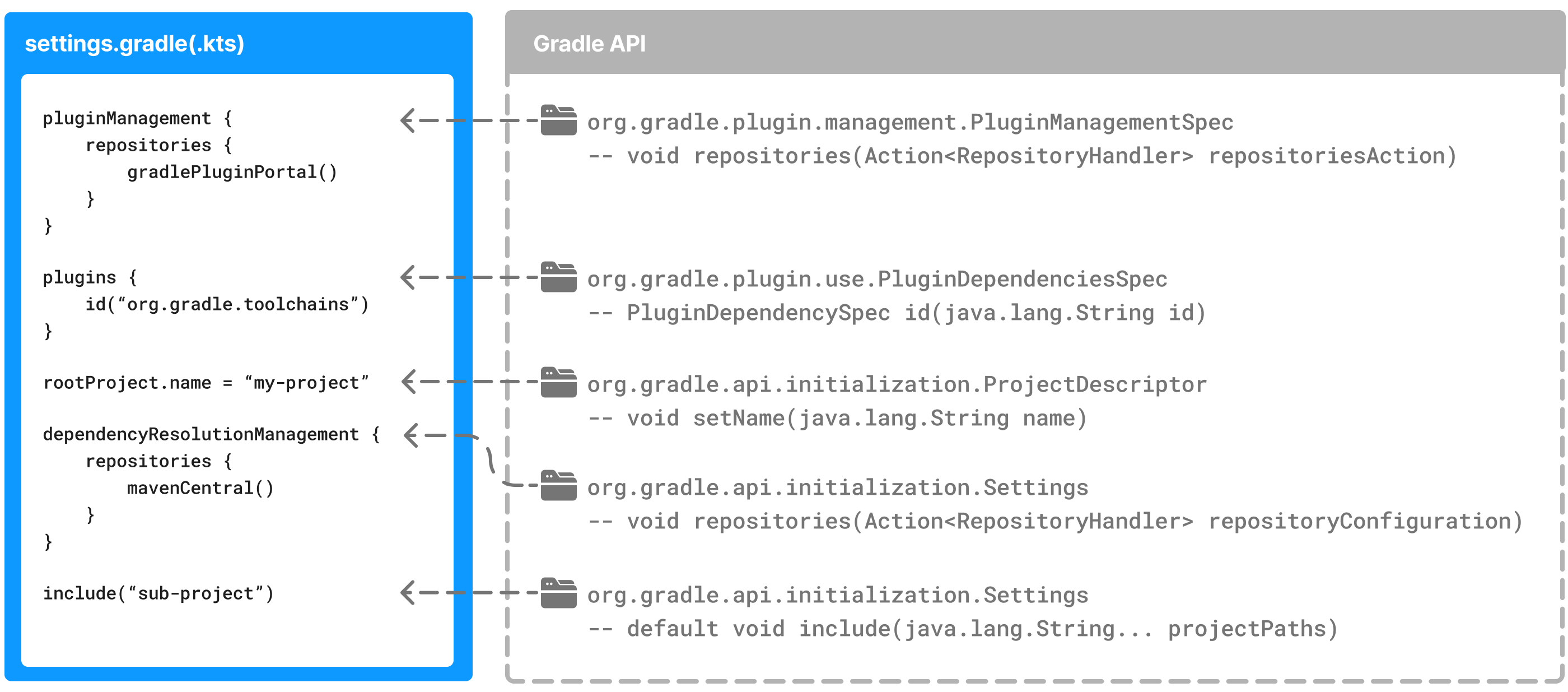
Early in the Gradle Build lifecycle, the initialization phase finds the settings file in your project root directory.
When the settings file settings.gradle(.kts)
is found, Gradle instantiates a Settings
object.
One of the purposes of the Settings
object is to allow you to declare all the projects to be included in the build.
Settings Scripts
The settings script is either a settings.gradle
file in Groovy or a settings.gradle.kts
file in Kotlin.
Before Gradle assembles the projects for a build, it creates a Settings
instance and executes the settings file against it.

As the settings script executes, it configures this Settings
.
Therefore, the settings file defines the Settings
object.
There is a one-to-one correspondence between a Settings instance and a settings.gradle(.kts) file.
|
The Settings
Object
The Settings
object is part of the Gradle API.
Many top-level properties and blocks in a settings script are part of the Settings API.
For example, we can set the root project name in the settings script using the Settings.rootProject
property:
settings.rootProject.name = "root"
Which is usually shortened to:
rootProject.name = "root"
Standard Settings
properties
The Settings
object exposes a standard set of properties in your settings script.
The following table lists a few commonly used properties:
Name | Description |
---|---|
|
The build cache configuration. |
|
The container of plugins that have been applied to the settings. |
|
The root directory of the build. The root directory is the project directory of the root project. |
|
The root project of the build. |
|
Returns this settings object. |
The following table lists a few commonly used methods:
Name | Description |
---|---|
|
Adds the given projects to the build. |
|
Includes a build at the specified path to the composite build. |
Settings Script structure
A Settings script is a series of method calls to the Gradle API that often use { … }
, a special shortcut in both the Groovy and Kotlin languages.
A { }
block is called a lambda in Kotlin or a closure in Groovy.
Simply put, the plugins{ }
block is a method invocation in which a Kotlin lambda object or Groovy closure object is passed as the argument.
It is the short form for:
plugins(function() {
id("plugin")
})
Blocks are mapped to Gradle API methods.
The code inside the function is executed against a this
object called a receiver in Kotlin lambda and a delegate in Groovy closure.
Gradle determines the correct this
object and invokes the correct corresponding method.
The this
of the method invocation id("plugin")
object is of type PluginDependenciesSpec
.
The settings file is composed of Gradle API calls built on top of the DSLs. Gradle executes the script line by line, top to bottom.
Let’s take a look at an example and break it down:
pluginManagement { (1)
repositories {
gradlePluginPortal()
google()
}
}
plugins { (2)
id("org.gradle.toolchains.fake") version "0.6.0"
}
rootProject.name = "root-project" (3)
dependencyResolutionManagement { (4)
repositories {
mavenCentral()
}
}
include("sub-project-a") (5)
include("sub-project-b")
include("sub-project-c")
1 | Define the location of plugins |
2 | Apply plugins. |
3 | Define the root project name. |
4 | Define build-wide repositories. |
5 | Add subprojects to the build. |
pluginManagement { (1)
repositories {
gradlePluginPortal()
google()
}
}
plugins { (2)
id 'org.gradle.toolchains.fake' version '0.6.0'
}
rootProject.name = 'root-project' (3)
dependencyResolutionManagement { (4)
repositories {
mavenCentral()
}
}
include('sub-project-a') (5)
include('sub-project-b')
include('sub-project-c')
1 | Define the location of plugins. |
2 | Apply plugins. |
3 | Define the root project name. |
4 | Define build-wide repositories. |
5 | Add subprojects to the build. |
1. Define the location of plugins
The settings file can optionally define the plugins your project uses with pluginManagement
, including binary repositories such as the Gradle Plugin Portal or other Gradle builds using includeBuild
:
pluginManagement {
repositories {
gradlePluginPortal()
google()
}
}
You can also include plugins and plugin dependency resolution strategies in this block.
2. Apply plugins
The settings file can optionally declare the plugins that may be applied later, which can add shared configuration among several builds / subprojects:
Plugins applied to the settings only affect the Settings
object.
plugins {
id("org.gradle.toolchains.fake") version "0.6.0"
}
This is typically used to ensure that all subprojects use the same plugin version.
3. Define the root project name
The settings file defines your project name using the rootProject.name
property:
rootProject.name = "root-project"
There is only one root project per build.
4. Define build-wide repositories
The settings file can optionally define the locations of components your project relies on (as well as how to resolve them) using repositories
such as binary repositories like Maven Central and/or other Gradle builds using includeBuild
:
dependencyResolutionManagement {
repositories {
mavenCentral()
}
}
You can also include version catalogs in this section.
5. Add subprojects to the build
The settings file defines the structure of the project by adding all the subprojects using the include
statement:
include("app")
include("business-logic")
include("data-model")
Settings File Scripting
There are many more properties and methods on the Settings
object that you can use to configure your build.
It’s important to remember that while many Gradle scripts are typically written in short Groovy or Kotlin syntax, every item in the settings script is essentially invoking a method on the Settings
object in the Gradle API:
include("app")
Is actually:
settings.include("app")
Additionally, the full power of the Groovy and Kotlin languages is available to you.
For example, instead of using include
many times to add subprojects, you can iterate over the list of directories in the project root folder and include them automatically:
rootDir.listFiles().filter { it.isDirectory && (new File(it, "build.gradle.kts").exists()) }.forEach {
include(it.name)
}
This type of logic should be developed in a plugin. |
Next Step: Learn how to write Build scripts >>