Part 1: Initializing the Project
- Step 0. Before you Begin
- Step 1. Initializing the Project
- Step 2. Understanding the Gradle Wrapper
- Step 3. Invoking the Gradle Wrapper
- Step 4. Understanding Gradle’s Project Structure
- Step 5. Viewing Gradle files in an IDE
- Step 6. Understanding the Settings file
- Step 7. Understanding the Build script
Learn the basics of Gradle by creating a Java app using Gradle init.
Step 0. Before you Begin
-
Make sure you have Gradle installed.
-
Install IntelliJ IDEA. The Community Edition is a free version of IntelliJ IDEA.
Step 1. Initializing the Project
To test the Gradle installation, run Gradle from the command-line:
$ gradle
Welcome to Gradle 8.6.
Directory '/' does not contain a Gradle build.
To create a new build in this directory, run gradle init
If Gradle is not installed, please refer to the installation section.
Create a new directory called tutorial
and cd
into it:
$ mkdir tutorial
$ cd tutorial
Run gradle init
with the following parameters to generate a Java application:
$ gradle init --type java-application --dsl kotlin
$ gradle init --type java-application --dsl groovy
Select defaults for any additional prompts.
In this tutorial, all examples are macOS based. |
When you are done, the directory should look as follows:
.
├── .gradle (1)
│ ├── libs.version.toml (2)
│ └── ⋮
├── gradle (3)
│ └── wrapper
├── gradlew (4)
├── gradlew.bat (5)
├── settings.gradle.kts (6)
├── app (7)
│ ├── build.gradle.kts
│ └── src
└── ⋮ (8)
1 | Project-specific cache directory generated by Gradle. |
2 | The version catalog which defines a set of versions for dependencies in a central location. |
3 | Contains the JAR file and configuration of the Gradle Wrapper. |
4 | macOS and Linux script for executing builds using the Gradle Wrapper. |
5 | Windows script for executing builds using the Gradle Wrapper. |
6 | The project’s settings file where the list of subprojects is defined. |
7 | The source code and build configuration for the Java app. |
8 | Some additional Git files may be present such as .gitignore . |
.
├── .gradle (1)
│ ├── libs.version.toml (2)
│ └── ⋮
├── gradle (3)
│ └── wrapper
├── gradlew (4)
├── gradlew.bat (5)
├── settings.gradle (6)
├── app (7)
│ ├── build.gradle
│ └── src
└── ⋮ (8)
1 | Project-specific cache directory generated by Gradle. |
2 | The version catalog which defines a set of versions for dependencies in a central location. |
3 | Contains the JAR file and configuration of the Gradle Wrapper. |
4 | macOS and Linux script for executing builds using the Gradle Wrapper. |
5 | Windows script for executing builds using the Gradle Wrapper. |
6 | The project’s settings file where the list of subprojects is defined. |
7 | The source code and build configuration for the Java app. |
8 | Some additional Git files may be present such as .gitignore . |
Step 2. Understanding the Gradle Wrapper
The Gradle Wrapper is the preferred way of starting a Gradle build. The Wrapper downloads (if needed) and then invokes a specific version of Gradle declared in the build.
In your newly created project, take a look at the files used by the Gradle Wrapper first. It consists of a shell script for macOS and Linux and a batch script for Windows .
These scripts allow you to run a Gradle build without requiring that Gradle be installed on your system. It also helps ensure that the same version of Gradle is used for builds by different developers and between local and CI machines.
From now on, you will never invoke Gradle directly; instead, you will use the Gradle wrapper.
Step 3. Invoking the Gradle Wrapper
Use the wrapper by entering the following command:
$ ./gradlew build
In Windows, the command is:
$ .\gradlew.bat build
The first time you run the wrapper, it downloads and caches the Gradle binaries if they are not already installed on your machine.
The Gradle Wrapper is designed to be committed to source control so that anyone can build the project without having to first install and configure a specific version of Gradle.
In this case, we invoked Gradle through the wrapper to build our project, so we can see that the app
directory now includes a new build
folder:
$ cd app
$ ls -al
drwxr-xr-x 10 gradle-user staff 320 May 24 18:07 build
-rw-r--r-- 1 gradle-user staff 862 May 24 17:45 build.gradle.kts
drwxr-xr-x 4 gradle-user staff 128 May 24 17:45 src
drwxr-xr-x 10 gradle-user staff 320 May 24 18:07 build
-rw-r--r-- 1 gradle-user staff 862 May 24 17:45 build.gradle
drwxr-xr-x 4 gradle-user staff 128 May 24 17:45 src
All the files generated by the build process go into the build
directory unless otherwise specified.
Step 4. Understanding Gradle’s Project Structure
Let’s take a look at a standard Gradle project structure and compare it to our tutorial project:
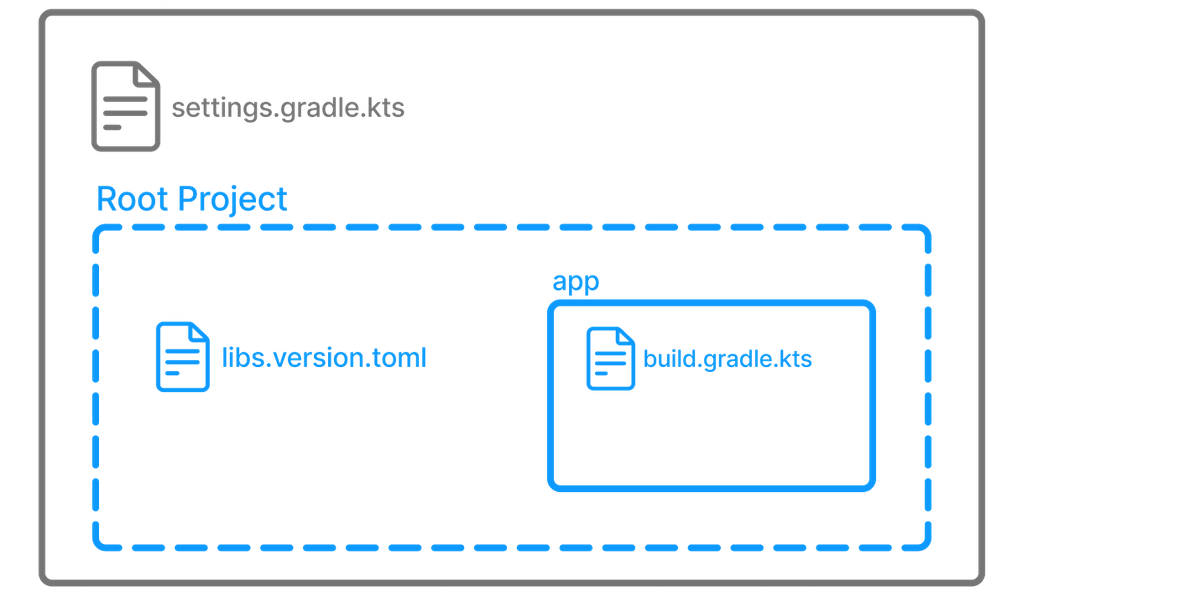
A build contains:
-
A top level
settings.gradle(.kts)
file. -
A root project.
-
One or more subprojects, each with its own
build.gradle(.kts)
file.
Some builds may contain a build.gradle(.kts)
file in the root project but this is NOT recommended.
The libs.version.toml
file is a version catalog used for dependency management which you will learn about in a subsequent section of the tutorial.
In this tutorial:
-
The root project is called tutorial and is defined with
rootProject.name = "tutorial"
in thesettings.gradle
file. -
The subproject is called app and is defined with
include("app")
in thesettings.gradle
file.
The root project can be in the top-level directory or have its own directory.
A build:
-
Represents a bundle of related software that you can build, test, and/or release together.
-
Can optionally include other builds (i.e. additional software such as libraries, plugins, build-time tools, etc).
A project:
-
Represents a single piece of your architecture - a library, an app, a Gradle plugin, etc.
-
Can optionally contain other projects.
Step 5. Viewing Gradle files in an IDE
Open the project in IntelliJ IDEA by double-clicking on the settings.gradle.kts
file in the tutorial
directory.
For Groovy DSL users, you may need to select the IntelliJ IDEA app when you click on the settings.gradle
file:
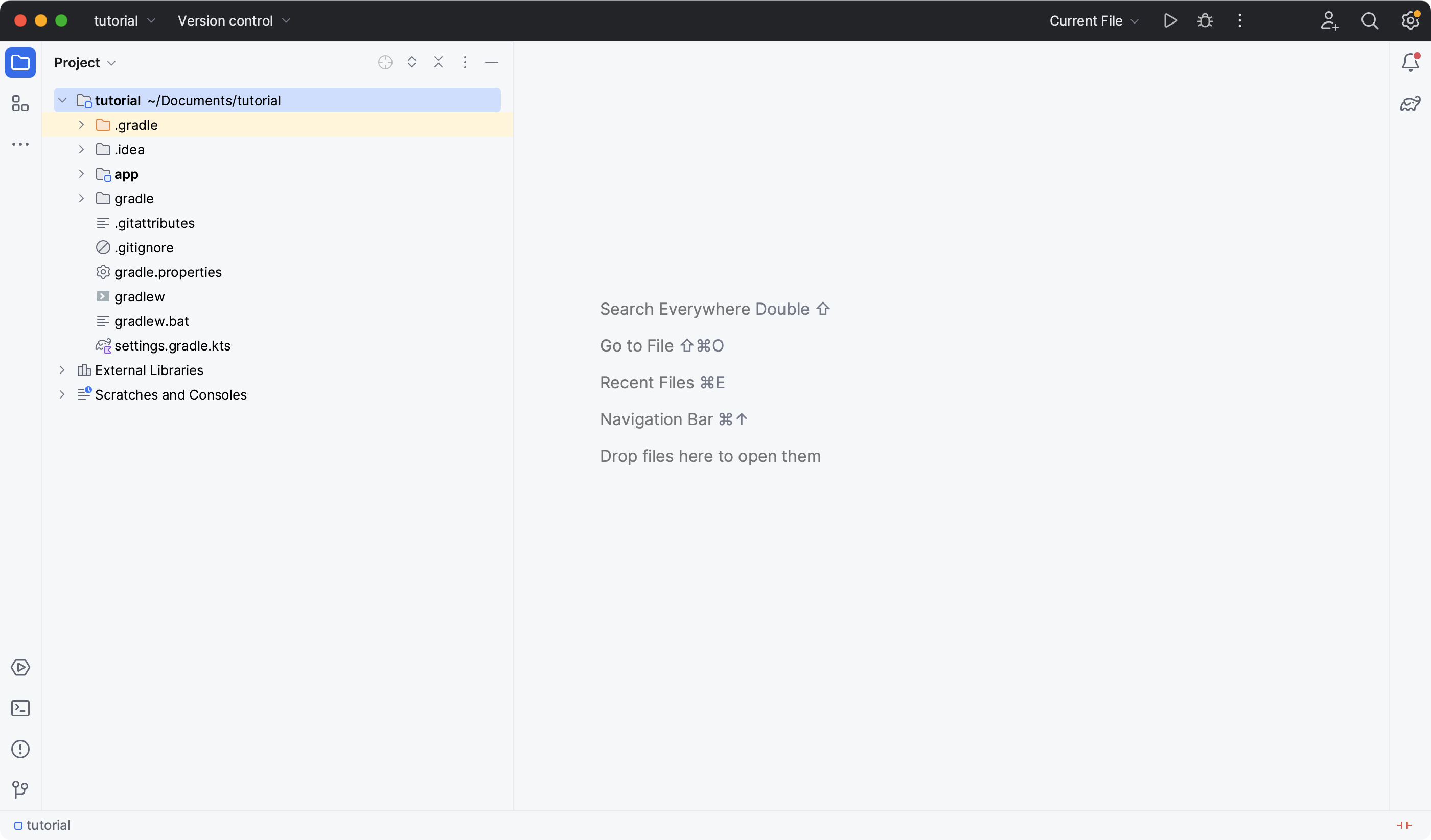
Open the settings.gradle(.kts)
and build.gradle(.kts)
files in the IDE:
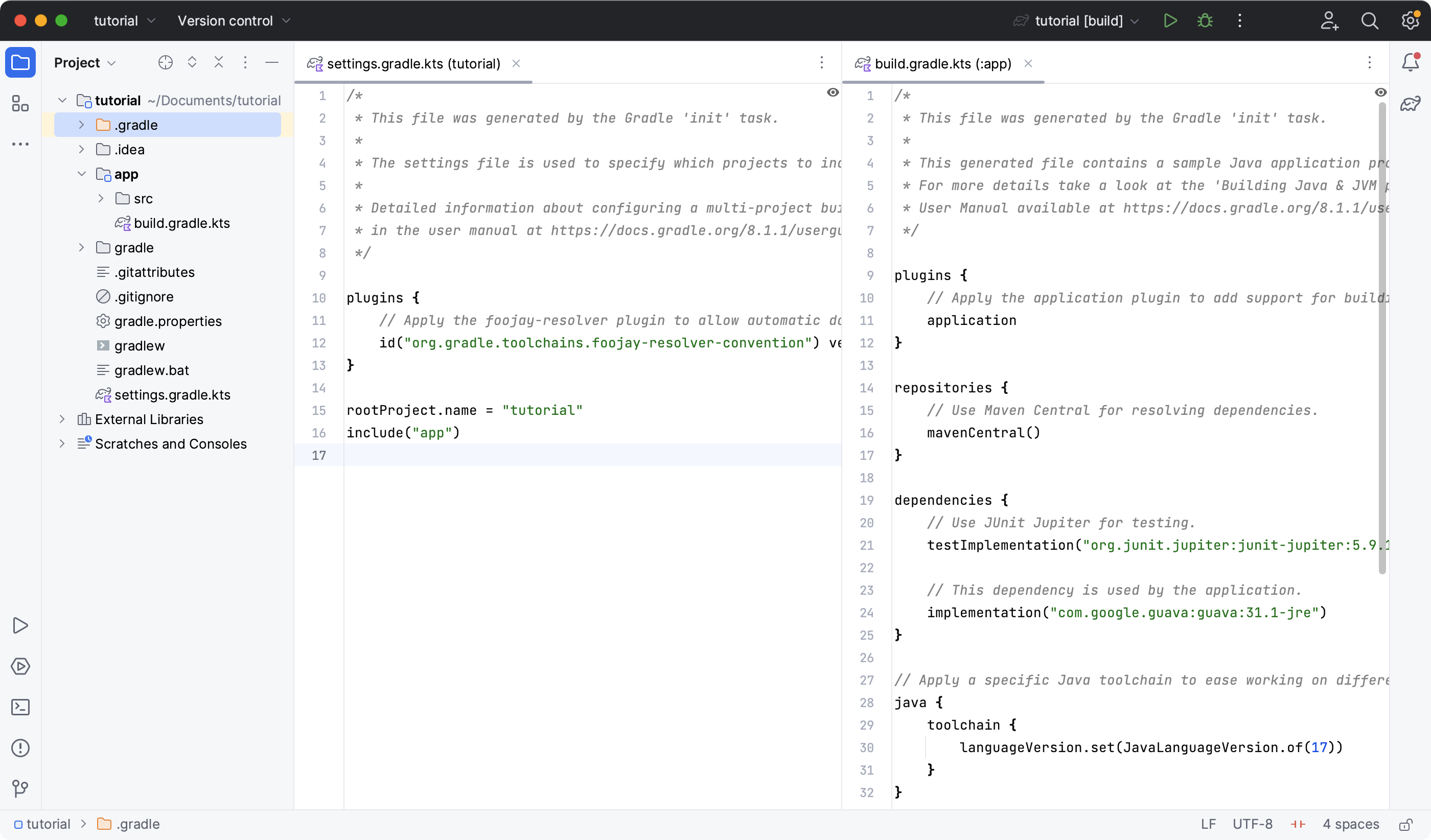
Step 6. Understanding the Settings file
A project is composed of one or more subprojects (sometimes called modules).
Gradle reads the settings.gradle(.kts)
file to figure out which subprojects comprise a project build.
Take a look at the file in your project:
plugins {
// Apply the foojay-resolver plugin to allow automatic download of JDKs
id("org.gradle.toolchains.foojay-resolver-convention") version "0.7.0"
}
rootProject.name = "tutorial"
include("app")
plugins {
// Apply the foojay-resolver plugin to allow automatic download of JDKs
id 'org.gradle.toolchains.foojay-resolver-convention' version '0.7.0'
}
rootProject.name = 'tutorial'
include('app')
The tutorial
root project includes the app
subproject.
The presence of the include
call turns the app
directory into a subproject.
Step 7. Understanding the Build script
Each subproject contains its own build.gradle(.kts)
file.
The build.gradle(.kts)
file is the core component of the build process and defines the tasks necessary to build the project.
The build.gradle(.kts)
file is read and executed by Gradle.
Take a closer look at the build file in your app
subproject (under the app
directory):
plugins {
// Apply the application plugin to add support for building a CLI application in Java.
application
}
repositories {
// Use Maven Central for resolving dependencies.
mavenCentral()
}
dependencies {
// Use JUnit Jupiter for testing.
testImplementation(libs.junit.jupiter)
testRuntimeOnly("org.junit.platform:junit-platform-launcher")
// This dependency is used by the application.
implementation(libs.guava)
}
// Apply a specific Java toolchain to ease working on different environments.
java {
toolchain {
languageVersion.set(JavaLanguageVersion.of(11))
}
}
application {
// Define the main class for the application.
mainClass.set("running.tutorial.kotlin.App")
}
tasks.named<Test>("test") {
// Use JUnit Platform for unit tests.
useJUnitPlatform()
}
plugins {
// Apply the application plugin to add support for building a CLI application in Java.
id 'application'
}
repositories {
// Use Maven Central for resolving dependencies.
mavenCentral()
}
dependencies {
// Use JUnit Jupiter for testing.
testImplementation libs.junit.jupiter
testRuntimeOnly 'org.junit.platform:junit-platform-launcher'
// This dependency is used by the application.
implementation libs.guava
}
// Apply a specific Java toolchain to ease working on different environments.
java {
toolchain {
languageVersion = JavaLanguageVersion.of(11)
}
}
application {
// Define the main class for the application.
mainClass = 'running.tutorial.groovy.App'
}
tasks.named('test') {
// Use JUnit Platform for unit tests.
useJUnitPlatform()
}
This build script lets Gradle know which dependencies and plugins the app
subproject is using and where to find them.
We will look at this in more detail in the following sections.
Next Step: Running Tasks >>