Plugin Basics
Gradle is built on a plugin system. Gradle itself is primarily composed of infrastructure, such as a sophisticated dependency resolution engine. The rest of its functionality comes from plugins.
A plugin is a piece of software that provides additional functionality to the Gradle build system.
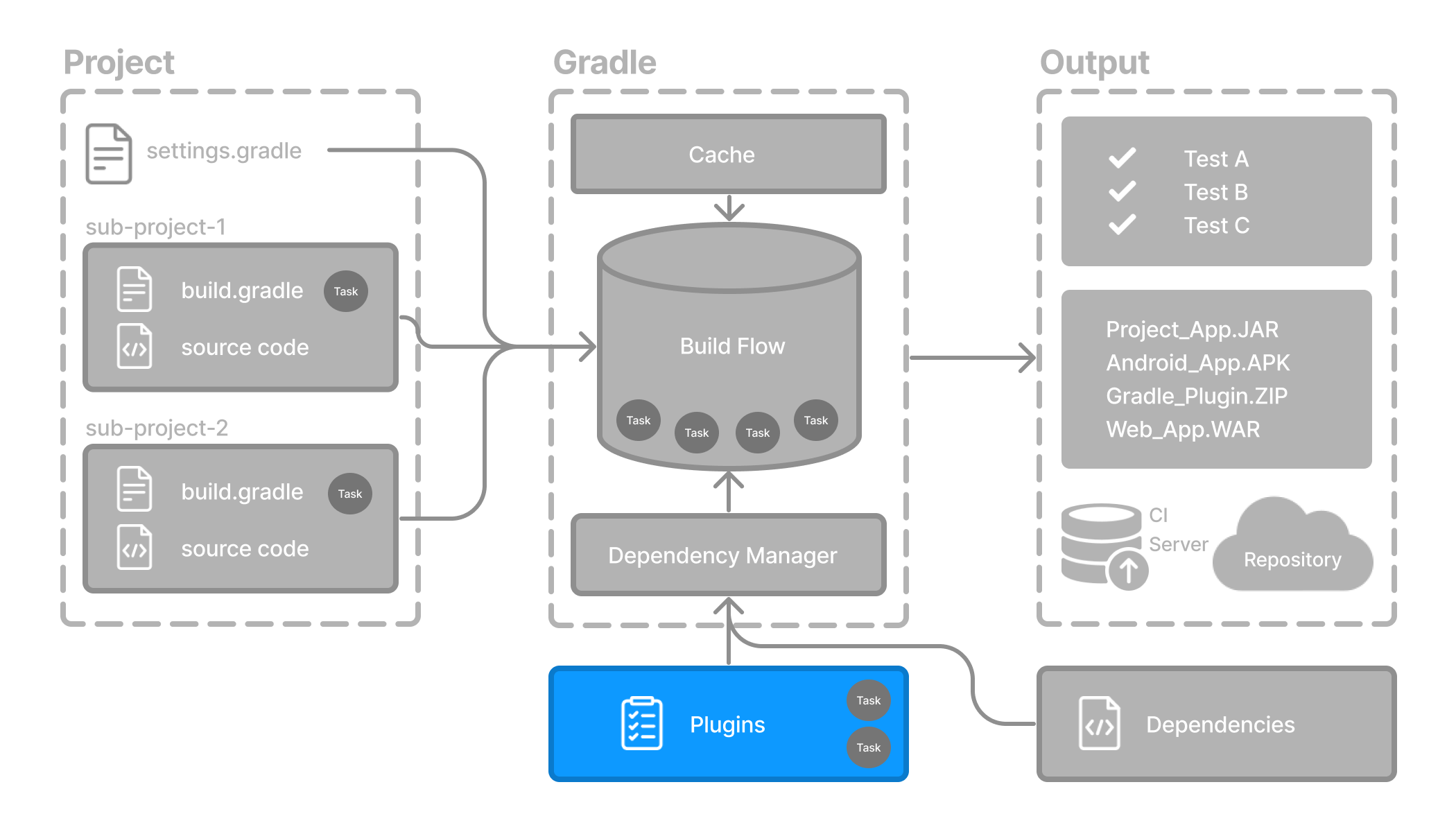
Plugins can be applied to a Gradle build script to add new tasks, configurations, or other build-related capabilities:
- The Java Library Plugin -
java-library
-
Used to define and build Java libraries. It compiles Java source code with the
compileJava
task, generates Javadoc with thejavadoc
task, and packages the compiled classes into a JAR file with thejar
task. - The Google Services Gradle Plugin -
com.google.gms:google-services
-
Enables Google APIs and Firebase services in your Android application with a configuration block called
googleServices{}
and a task calledgenerateReleaseAssets
. - The Gradle Bintray Plugin -
com.jfrog.bintray
-
Allows you to publish artifacts to Bintray by configuring the plugin using the
bintray{}
block.
Plugin distribution
Plugins are distributed in three ways:
-
Core plugins - Gradle develops and maintains a set of Core Plugins.
-
Community plugins - Gradle’s community shares plugins via the Gradle Plugin Portal.
-
Local plugins - Gradle enables users to create custom plugins using APIs.
Applying plugins
Applying a plugin to a project allows the plugin to extend the project’s capabilities.
You apply plugins in the build script using a plugin id (a globally unique identifier / name) and a version:
plugins {
id «plugin id» version «plugin version»
}
1. Core plugins
Gradle Core plugins are a set of plugins that are included in the Gradle distribution itself. These plugins provide essential functionality for building and managing projects.
Some examples of core plugins include:
-
java: Provides support for building Java projects.
-
groovy: Adds support for compiling and testing Groovy source files.
-
ear: Adds support for building EAR files for enterprise applications.
Core plugins are unique in that they provide short names, such as java
for the core JavaPlugin, when applied in build scripts.
They also do not require versions.
To apply the java
plugin to a project:
plugins {
id("java")
}
There are many Gradle Core Plugins users can take advantage of.
2. Community plugins
Community plugins are plugins developed by the Gradle community, rather than being part of the core Gradle distribution. These plugins provide additional functionality that may be specific to certain use cases or technologies.
The Spring Boot Gradle plugin packages executable JAR or WAR archives, and runs Spring Boot Java applications.
To apply the org.springframework.boot
plugin to a project:
plugins {
id("org.springframework.boot") version "3.1.5"
}
Community plugins can be published at the Gradle Plugin Portal, where other Gradle users can easily discover and use them.
3. Local plugins
Custom or local plugins are developed and used within a specific project or organization. These plugins are not shared publicly and are tailored to the specific needs of the project or organization.
Local plugins can encapsulate common build logic, provide integrations with internal systems or tools, or abstract complex functionality into reusable components.
Gradle provides users with the ability to develop custom plugins using APIs. To create your own plugin, you’ll typically follow these steps:
-
Define the plugin class: create a new class that implements the
Plugin<Project>
interface.// Define a 'HelloPlugin' plugin class HelloPlugin : Plugin<Project> { override fun apply(project: Project) { // Define the 'hello' task val helloTask = project.tasks.register("hello") { doLast { println("Hello, Gradle!") } } } }
-
Build and optionally publish your plugin: generate a JAR file containing your plugin code and optionally publish this JAR to a repository (local or remote) to be used in other projects.
// Publish the plugin plugins { `maven-publish` } publishing { publications { create<MavenPublication>("mavenJava") { from(components["java"]) } } repositories { mavenLocal() } }
-
Apply your plugin: when you want to use the plugin, include the plugin ID and version in the
plugins{}
block of the build file.// Apply the plugin plugins { id("com.example.hello") version "1.0" }
Consult the Plugin development chapter to learn more.
Next Step: Learn about Incremental Builds and Build Caching >>